Reading time: 11 minutes.
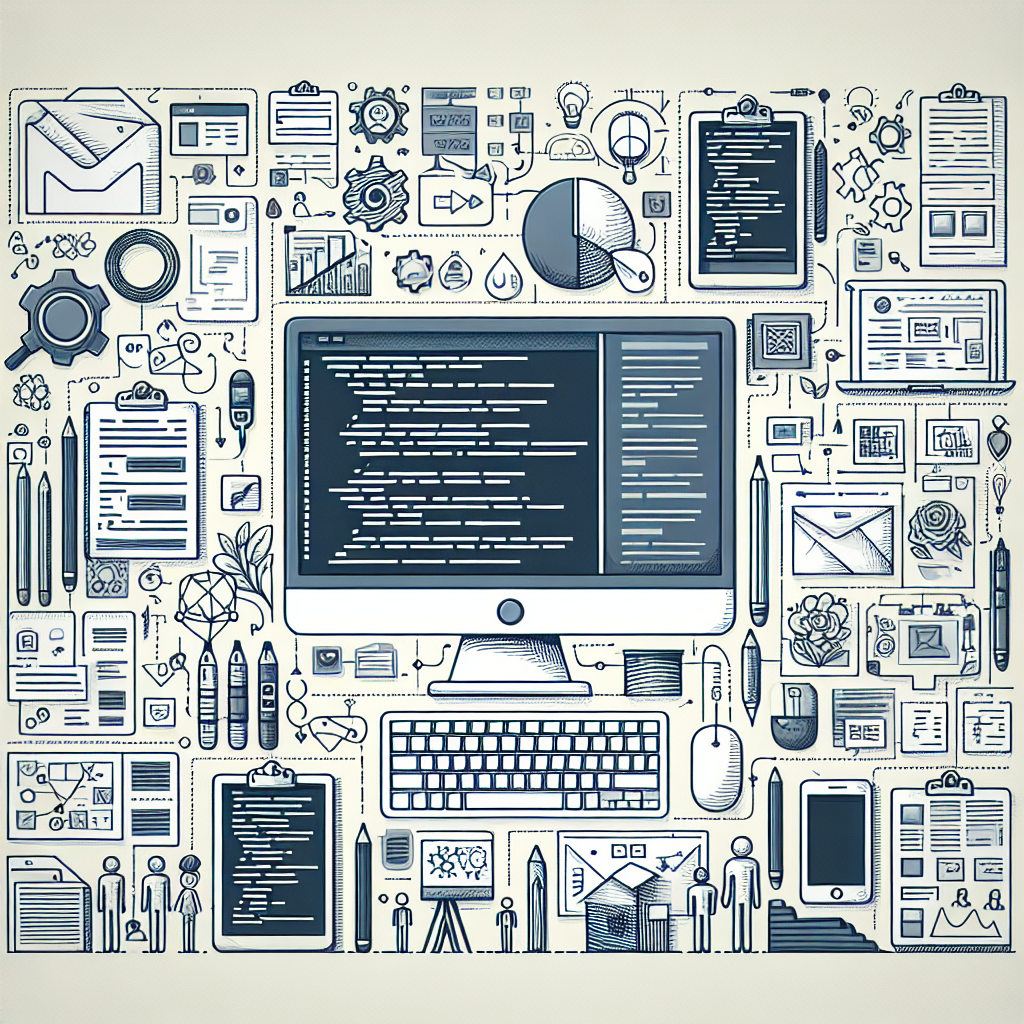
My Journey in Front End Web Development
Introduction to Front End Development
Embarking on a journey in front end web development, I initially found myself intrigued by the seamless interaction between design and functionality. Front end development focuses on what users see and interact with within their web browsers. The core languages that make this possible are HTML, CSS, and JavaScript. Everything from the layout of a website to its aesthetic appeal and interactive features stems from effective use of these technologies.
I began with learning HTML to structure web content, moved on to CSS for styling, and finally dove into JavaScript to add dynamic behavior. While these technologies provided the foundation, I quickly realized the importance of additional tools and frameworks. Understanding web development frameworks, and embracing responsive design principles were pivotal in enhancing my skills.
Initial Challenges Faced
The initial challenges in my front end web development journey were numerous. Building a user-friendly interface required more than just knowledge of basic coding. Here are some hurdles I encountered and overcame:
Understanding the Document Object Model (DOM):
Manipulating the DOM efficiently was a hurdle. Learning how to interact with various elements dynamically using JavaScript was a steep learning curve.Mastering Responsive Design:
Ensuring that websites function well on different devices and screen sizes was essential. Adopting responsive web development techniques, like using media queries and flexible grid systems, was crucial.Cross-Browser Compatibility:
Ensuring that web applications render consistently across different browsers was a persistent challenge. Each browser has its own quirks, making it necessary to test extensively.Performance Optimization:
Websites need to load quickly and run efficiently. Learning to optimize HTML, CSS, and JavaScript, as well as understanding tools for performance testing, was essential.
Challenge | Description |
---|---|
Understanding the DOM | Dynamically interacting with elements using JavaScript |
Mastering Responsive Design | Adopting techniques for various devices and screen sizes |
Cross-Browser Compatibility | Ensuring consistent rendering across browsers |
Performance Optimization | Enhancing load speeds and run-time efficiency |
These early challenges taught me valuable lessons about the importance of clean and efficient code, and set the foundation for further learning. The experience of resolving these issues reinforced my dedication to continuous learning and improvement. For those new to the field, aspiring to enhance their skills through a web development bootcamp or obtaining relevant web development certifications can be highly beneficial.
By reflecting on the fundamentals of front end web development and addressing initial challenges, I laid a strong foundation for further exploration and growth in this dynamic field.
Essential Tools and Technologies
Embarking on my front end web development journey, I quickly learned the importance of mastering the essential tools and technologies. These foundational elements are critical in building effective and visually appealing web applications.
HTML, CSS, and JavaScript
HTML (Hypertext Markup Language), CSS (Cascading Style Sheets), and JavaScript are the core elements of front end development. HTML structures the content, CSS styles it, and JavaScript adds interactivity.
- HTML
- Defines the structure of web pages
- Uses tags to organize content
- CSS
- Controls the presentation and layout
- Employs properties like color, margin, and padding
- JavaScript
- Adds dynamic behavior and interactivity
- Utilizes functions, events, and DOM manipulation
Language | Purpose | Example |
---|---|---|
HTML | Structure | <h1>Hello World</h1> |
CSS | Style | h1 { color: blue; } |
JavaScript | Behavior | document.querySelector('h1').onclick = function() { alert('Hello World'); } |
Understanding the interplay between these languages is essential for any web developer. For those exploring more on the role of these elements in earning potential, see our article on web development salary.
Responsive Design and Frameworks
Responsive design ensures that web content adjusts seamlessly across different devices, providing an optimal viewing experience. Frameworks and libraries assist in implementing responsive design by offering pre-built components and grid systems.
- Responsive Design
- Uses flexible layouts and media queries
- Adapts to various screen sizes and orientations
Screen Size | Device | Example Media Query |
---|---|---|
Small | Smartphone | @media only screen and (max-width: 600px) |
Medium | Tablet | @media only screen and (max-width: 768px) |
Large | Desktop | @media only screen and (min-width: 1024px) |
- Frameworks
- Provide a foundation of pre-written code
- Enhance efficiency and consistency
Popular front end frameworks include Bootstrap, Foundation, and Tailwind CSS. These tools greatly simplify the process of creating responsive designs. For further insights on frameworks, check out our article on web development frameworks.
By mastering these essential tools and technologies, I could efficiently create engaging and responsive web applications. As I continued to learn, the importance of implementing clean and efficient code became evident. For those interested in further developing their skills, consider joining a web development bootcamp.
Lessons Learned in Coding
Over my journey in front end web development, I’ve gained numerous insights that have significantly improved my coding skills. Two key areas that have had a profound impact on my work are understanding DOM manipulation and the importance of writing clean and efficient code.
Understanding DOM Manipulation
DOM (Document Object Model) manipulation is an essential skill for any front end developer. It involves interacting with the structure of a web page, allowing for dynamic content updates without requiring a page reload. Early on, I realized that mastering this concept was crucial for creating interactive and responsive web applications.
I found that using native JavaScript methods like getElementById
and querySelector
were fundamental. These methods allowed me to access and manipulate HTML elements directly. Understanding how to work with event listeners, such as addEventListener
, enabled me to make my web pages more interactive.
Here’s a simple example of DOM manipulation using JavaScript:
document.getElementById('myButton').addEventListener('click', function() {
document.getElementById('myText').innerHTML = 'Hello, World!';
});
This snippet demonstrates how clicking a button can change the content of a text element. Learning these basics paved the way for more complex interactions and functionalities in my projects.
Importance of Clean and Efficient Code
One of the most valuable lessons I learned was the importance of writing clean and efficient code. Clean code is not just about making the code look good; it’s about making it understandable and maintainable for others and for my future self.
Key practices that I adopted include:
- Consistent naming conventions
- Modular code structure
- Avoiding deep nesting
- Commenting and documentation
Adhering to these practices made my code more readable and easier to debug. Moreover, I discovered that modular code structure, such as using functions and modules, allowed for better code reuse and maintainability.
Here’s a comparison table to highlight the benefits of clean and efficient code:
Aspect | Dirty Code | Clean Code |
---|---|---|
Readability | Low | High |
Maintainability | Difficult | Easy |
Debugging | Time-consuming | Efficient |
Reusability | Poor | Excellent |
Writing efficient code also meant optimizing my JavaScript logic to improve performance. For example, minimizing DOM manipulations by batching updates and reducing unnecessary reflows and repaints led to much smoother user experiences.
By focusing on clean and efficient coding practices, I could develop high-quality web applications that performed well and were easy to maintain. For more insights on front end development practices, check out our web development tools and responsive web development articles.
User Experience and Design Principles
User experience (UX) plays a critical role in front end web development. My journey in this field has taught me that creating a seamless, intuitive experience is just as important as writing clean, efficient code.
Usability and Accessibility
Usability and accessibility are cornerstone principles in delivering exceptional user experiences. Usability focuses on how easily users can interact with a website, while accessibility ensures that people with disabilities can use the site effectively.
Usability
To improve usability, I prioritize:
- Simple Navigation: Users should effortlessly find what they are looking for. I use clear labels and logical structures to guide them.
- Consistent Design: Maintaining a uniform style across pages helps users understand how to interact with the site.
- Fast Load Times: Speed is crucial. I optimize images and scripts to keep load times minimal.
Accessibility
Ensuring accessibility involves several key practices:
- Semantic HTML: Utilizing appropriate HTML tags (like
<header>
,<main>
, and<footer>
) makes the content more understandable for screen readers. - Alt Text for Images: I always include descriptive alt text for images to assist visually impaired users.
- Keyboard Navigation: Ensuring that all interactive elements are reachable via keyboard helps users who cannot use a mouse.
For a deeper dive into how to make sites more accessible, see our article on web development services.
Design Trends and Best Practices
Staying updated with current design trends is essential to keep the website appealing and effective.
Design Trends
Here are some trends I consider:
- Minimalist Design: Simple layouts with ample white space prevent user overload.
- Microinteractions: Small, subtle animations can guide users and provide feedback, enhancing the overall experience.
- Dark Mode: This offers an alternative color scheme that can be easier on the eyes and save battery life on OLED screens.
Best Practices
Following best practices ensures the design remains effective and user-centric:
- Mobile-First Design: Given the increase in mobile usage, designing for mobile devices first ensures a responsive layout that works across all platforms. Learn more about this in our article on responsive web development.
- Consistent Branding: Consistency in colors, fonts, and visual styles across the site helps build brand identity.
- User Testing: Regular testing helps identify and fix usability issues before they become significant problems.
By focusing on usability, accessibility, and staying current with design trends, I’ve found that the overall user experience of a website can be significantly enhanced. For those looking to further their knowledge, consider exploring web development certifications to gain formal training in these crucial areas.
Working with APIs and Integrations
APIs (Application Programming Interfaces) play a critical role in front-end web development, enabling developers to fetch and manipulate data from various sources. Understanding how to work with APIs can significantly enhance the functionality and user experience of a web application.
Fetching Data from APIs
Fetching data from APIs involves making requests to external servers, retrieving the desired data, and displaying it on your webpage. In the beginning, I struggled with understanding how to make these requests and handle the responses effectively. However, with practice, I learned that using methods like fetch
and axios
simplifies the process.
To fetch data using the fetch
method, the basic syntax looks like this:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
// Process and display data
console.log(data);
})
.catch(error => console.error('Error:', error));
The fetch
method returns a promise that resolves to a response object. Calling .json()
on the response object parses the response into a JavaScript object. It’s crucial to handle errors appropriately to ensure a smooth user experience.
Handling Responses and Data Formats
Once data is fetched from an API, it often comes in formats such as JSON or XML. Understanding how to handle these responses is essential. JSON is the most commonly used format due to its simplicity and compatibility with JavaScript.
Here is a table illustrating common data formats and their characteristics:
Data Format | Description | Usage |
---|---|---|
JSON | Lightweight, easy-to-parse | Web APIs, data interchange |
XML | Markup language, more complex | Configuration, data interchange |
CSV | Comma-separated values | Data export/import |
Handling a JSON response typically involves parsing the data and updating the DOM to display the retrieved information. Here’s a simple example:
fetch('https://api.example.com/users')
.then(response => response.json())
.then(users => {
const userList = document.getElementById('user-list');
users.forEach(user => {
let listItem = document.createElement('li');
listItem.textContent = `${user.name} - ${user.email}`;
userList.appendChild(listItem);
});
})
.catch(error => console.error('Error:', error));
In this example, the user data is fetched from the API, parsed, and then dynamically added to a list in the DOM.
Understanding how to fetch and handle data from APIs opens up a world of possibilities for front-end web developers. It allows for the integration of real-time data, enhances interactivity, and ultimately improves user satisfaction. For more insights on essential tools for web development, visit web development tools and explore various frameworks in web development frameworks.
Continuous Learning and Growth
In the ever-evolving field of front end web development, continuous learning and growth are essential. Staying updated with industry trends and constantly upskilling has been crucial in my development journey.
Staying Updated with Industry Trends
The world of web development evolves quickly, with new tools, frameworks, and best practices emerging regularly. Staying current with these trends ensures that my skills remain relevant and competitive. Here are some ways I stay updated:
- Reading Blogs and Articles: Blogs and articles from experienced developers provide valuable insights and updates on the latest industry trends.
- Participating in Online Communities: Forums and social media platforms dedicated to web development offer discussions, tips, and the latest news.
- Attending Webinars and Conferences: These events are great opportunities to learn from experts and network with other developers.
- Subscribing to Newsletters: Newsletters from trusted sources deliver the latest updates directly to my inbox.
- Following Web Development Channels: YouTube channels and podcasts focused on web development also provide valuable knowledge.
Resource Type | Example |
---|---|
Blogs and Articles | Smashing Magazine, CSS-Tricks |
Online Communities | Stack Overflow, Reddit |
Webinars/Conferences | React Conf, Chrome Dev Summit |
Newsletters | Frontend Focus, JavaScript Weekly |
Channels/Podcasts | Traversy Media, Syntax |
Exploring these resources ensures that I stay informed about the latest in responsive web development and other key areas in front end development.
Upskilling and Building Projects for Practice
Actively upskilling and working on projects is vital for practical learning and honing my front end web development skills. Here’s how I approach this:
- Taking Online Courses: Online courses offer structured learning paths and hands-on projects to build practical skills.
- Building Personal Projects: Applying new skills to personal projects helps bridge the gap between theory and practice.
- Contributing to Open Source: Working on open-source projects can enhance my coding skills and provide valuable experience.
- Joining a Coding Bootcamp: A web development bootcamp offers immersive learning experiences that are both intense and rewarding.
- Pursuing Certifications: Earning web development certifications validates my skills and increases my credibility.
Upskilling Method | Example |
---|---|
Online Courses | freeCodeCamp, Coursera |
Personal Projects | Portfolio site, JavaScript games |
Open Source Contribution | GitHub repositories |
Coding Bootcamps | Web Dev Bootcamp, Codecademy Pro |
Certifications | Google Developer Certification |
Frequent upskilling and project building not only improve my proficiency but also keep me engaged and motivated in my front end web development journey. This ongoing commitment to learning helps me stay at the forefront of the industry, ensuring that I deliver top-notch web development services.