Reading time: 10 minutes.
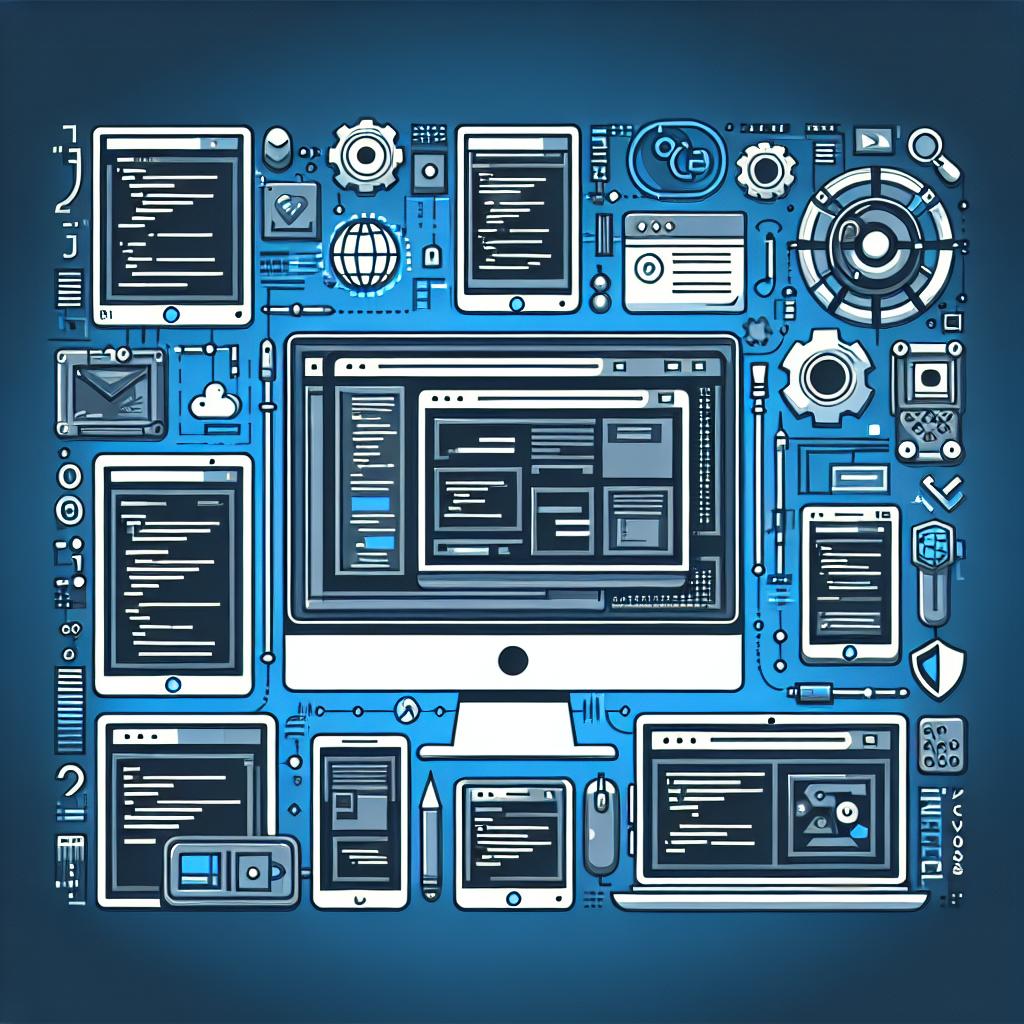
Introduction to Responsive Web Development
Importance of Responsive Design
Responsive web design is a fundamental aspect of modern web development. It ensures that a website’s layout and content adapt seamlessly to various screen sizes and devices. A responsive design provides an optimal viewing experience—whether on a desktop, a tablet, or a mobile device. This adaptability improves usability and keeps users engaged.
Key benefits of responsive design include:
- Enhanced user experience: A responsive site improves readability and navigation.
- SEO benefits: Search engines favor responsive websites, potentially boosting search rankings.
- Cost-effectiveness: A single responsive website means no need for separate mobile and desktop versions.
Responsive web development utilizes techniques like fluid grids, flexible images, and CSS media queries to create layouts that adapt to different screen sizes. For those interested in diving deeper, explore our guide on web development frameworks.
Evolution of Mobile Device Usage
Over the years, mobile device usage has skyrocketed, making it imperative for websites to be mobile-friendly. This increase in mobile browsing has reshaped how developers approach web design. Understanding the evolution of mobile device usage provides context for the importance of responsive web development.
Year | Global Mobile Traffic Percentage |
---|---|
2010 | 4% |
2013 | 17% |
2016 | 43% |
2019 | 53% |
2022 | 58% |
These numbers illustrate the growing prominence of mobile devices for internet access. Ignoring this trend could alienate a significant portion of the audience. Web developers should prioritize responsive design to ensure that users have a seamless experience across all devices.
Responsive web development isn’t just about making websites mobile-friendly; it’s about creating adaptable, flexible designs that respond to the needs of the user. For more insights on the tools and techniques involved in creating responsive websites, check our resources on web development tools.
Building a Responsive Website
Responsive web development is a vital aspect of modern web creation, ensuring that websites adapt seamlessly across various devices and screen sizes. In this section, I’ll delve into frameworks and libraries, as well as media queries and breakpoints, key components in building a responsive website.
Frameworks and Libraries
Frameworks and libraries provide pre-built, tested components and styles, streamlining the development process. They enable me to create responsive layouts efficiently, ensuring consistency across different devices.
Popular Frameworks:
- Bootstrap: Offers a comprehensive collection of HTML, CSS, and JS components.
- Foundation: Provides robust features, including a responsive grid system.
- Bulma: A modern CSS framework based on Flexbox.
Key Libraries:
- jQuery: Simplifies HTML DOM tree traversal and manipulation.
- React: A JavaScript library for building user interfaces.
- Vue.js: An approachable, versatile framework for building interactive UIs.
Using these frameworks and libraries not only speeds up development but also helps maintain cross-browser compatibility. For more information on frameworks, check out our article on web development frameworks, which provides insights into selecting the right one for your project.
Media Queries and Breakpoints
Media queries are a fundamental part of responsive web development. They allow me to apply CSS rules based on device characteristics such as screen width, height, resolution, and orientation. By defining breakpoints, I ensure that the design adapts gracefully to different screen sizes.
Example of Media Queries:
/* Default styles for mobile devices */
body {
font-size: 14px;
}
/* Styles for tablets (min-width: 768px) */
@media (min-width: 768px) {
body {
font-size: 16px;
}
}
/* Styles for desktops (min-width: 1024px) */
@media (min-width: 1024px) {
body {
font-size: 18px;
}
}
Common Breakpoints:
Device Type | Min Width (px) | Max Width (px) |
---|---|---|
Mobile | 320 | 767 |
Tablet | 768 | 1023 |
Desktop | 1024 | – |
By carefully selecting breakpoints, I ensure that the website’s layout, typography, and other elements adjust properly to offer an optimal viewing experience. Understanding how to implement media queries and breakpoints is crucial for any web developer aiming to enhance their skills in responsive web development.
For those new to web development or wanting to deepen their knowledge, consider enrolling in a web development bootcamp or exploring resources on web development tools.
In summary, leveraging frameworks, libraries, media queries, and breakpoints is essential for creating a responsive website that meets the needs of users across diverse devices. By following these best practices, I’m able to deliver an adaptable, user-friendly web experience.
Optimizing for Different Screen Sizes
Understanding how to optimize websites for different screen sizes is central to responsive web development. This section will guide you through the essentials of viewports and the specifics of designing for mobile, tablet, and desktop screens.
Understanding Viewports
Viewports are a critical concept in responsive web design. They refer to the visible area of a web page on a device’s screen. By configuring viewports properly, users can ensure that web content scales correctly across various devices.
The viewport is defined using the <meta>
tag in HTML. Here’s an example:
<meta name="viewport" content="width=device-width, initial-scale=1.0">
This tag instructs the browser on how to control the page’s dimensions and scaling. The parameters such as width=device-width
and initial-scale=1.0
are essential for achieving an optimal display.
Designing for Mobile, Tablet, and Desktop
Creating responsive designs involves considering the unique requirements of various devices. Here’s how I approach it:
Mobile
Mobile design focuses on simplicity and usability. Key aspects include:
- Prioritizing content: Displaying the most important information first.
- Using larger touch targets: Ensuring buttons and links are easily tappable.
- Simplified navigation: Using hamburger menus and other mobile-friendly navigation methods.
To help with the layout, I often rely on breakpoints in CSS, such as:
@media (max-width: 600px) {
body {
font-size: 16px;
}
}
Tablet
Tablet design requires a balance between mobile and desktop experiences. Consider:
- Adapting grid layouts: Ensuring content appears well-organized in columns.
- Scalable images: Using responsive images to fit different screen sizes.
- Enhanced navigation: Incorporating touch gestures for a better user experience.
An example of breakpoints for tablets:
@media (min-width: 601px) and (max-width: 900px) {
body {
font-size: 18px;
}
}
Desktop
Desktop design offers more space, enabling more complex layouts. Important elements include:
- Multi-column layout: Utilizing a grid system.
- Rich media content: Adding high-resolution images and videos.
- Comprehensive navigation: Using expanded menus and additional navigation options.
Breakpoint example for desktop:
@media (min-width: 901px) {
body {
font-size: 20px;
}
}
The following table summarizes common breakpoints for different devices:
Device | Min Width (px) | Max Width (px) |
---|---|---|
Mobile | 0 | 600 |
Tablet | 601 | 900 |
Desktop | 901 | None |
By understanding viewports and tailoring designs to specific screen sizes, I can create websites that offer a seamless user experience across all devices.
For more insights into topics like web development frameworks or web development tools, check out the related articles.
Performance and Speed Considerations
In responsive web development, performance and speed play crucial roles in ensuring a seamless user experience. Optimizing images and minifying code are essential techniques to improve load times and overall efficiency.
Image Optimization
Images often account for a significant portion of a webpage’s loading time. Optimizing them can drastically enhance performance. Here are key methods for image optimization:
- Compression: Reducing the file size of images without compromising quality. It’s vital to use compression algorithms that maintain image fidelity while decreasing size.
- Responsive Images: Serving different image sizes based on the user’s device to prevent unnecessary data usage. This can be achieved using the
<picture>
element andsrcset
attribute in HTML. - Format Selection: Choosing the right image format is essential. Formats like JPEG, PNG, and WebP each have their advantages depending on the use case.
Technique | Description | Example |
---|---|---|
Compression | Decrease file size without quality loss | JPEG, PNG |
Responsive Images | Serve various sizes for different devices | <picture> tag |
Format Selection | Use the best format for the situation | WebP for web use |
Implementing these strategies can effectively reduce loading times, improving user engagement and satisfaction.
Code Minification and Compression
Minifying and compressing code reduces file sizes and makes data transfer more efficient. These techniques are crucial for improving the speed of a responsive website.
- HTML Minification: Removing unnecessary characters like whitespace, comments, and line breaks. This process results in smaller HTML files, which load faster.
- CSS and JavaScript Minification: Similar to HTML minification, targeting CSS and JS files to eliminate all non-essential spaces and comments. Tools for this process help streamline files without affecting functionality.
- Code Compression: Using algorithms to compress files for faster transmission over the internet. Gzip is a commonly used method for compressing web files.
Technique | Description | Benefits |
---|---|---|
HTML Minification | Remove extra characters and comments | Smaller file sizes |
CSS/JS Minification | Eliminate whitespace and comments | Faster loading times |
Code Compression | Compress with algorithms like Gzip | Efficient data transfer |
By applying these optimization methods, developers can ensure their responsive websites perform well across all devices. Improving speed and performance enhances the overall user experience, making these techniques indispensable for any web developer. For more on optimizing responsive websites, explore our resources on web development tools and front end web development.
User Experience and Navigation
A crucial aspect of responsive web development is ensuring an optimal user experience (UX) across various devices. This entails designing navigation systems and touch interactions that cater to users on mobile, tablet, and desktop screens.
Mobile-Friendly Navigation
Mobile-friendly navigation is essential for responsive design. On smaller screens, traditional navigation menus can clutter the interface, making it difficult for users to find what they need. To combat this, consider implementing:
- Hamburger Menus: These condensed menus save space and provide a clean look. When tapped, they expand to reveal the navigation options.
- Sticky Navigation: Keeping the navigation bar fixed at the top improves accessibility, allowing users to access navigation options without scrolling back to the top.
- Dropdown Menus: Dropdowns help organize complex navigation systems without overwhelming the user.
Navigation Type | Description |
---|---|
Hamburger Menu | Collapses into a three-line icon |
Sticky Navigation | Stays at the top of the screen during scroll |
Dropdown Menus | Expands to show sub-menus when clicked |
For a detailed look at how different web development frameworks can assist in creating mobile-friendly navigation, visit our article on web development frameworks.
Touch Interactions and Gestures
Touch interactions and gestures are fundamental for enhancing the UX on touch devices such as smartphones and tablets. Incorporating intuitive touch features improves navigation and overall user satisfaction. Key aspects to consider:
- Tap Targets: Ensure buttons and links are large enough to be easily tapped, reducing user frustration and errors.
- Swipe Gestures: Implement swipe gestures for actions like navigating between pages or images. Swiping left or right can provide a seamless experience for users.
- Pinch-to-Zoom: Allow users to zoom in and out of images and text for better readability.
- Long Press: Use long press gestures for additional options or context menus.
Touch Gesture | Function |
---|---|
Tap | Select or activate an element |
Swipe | Navigate or reveal additional content |
Pinch-to-Zoom | Adjust size of images or text |
Long Press | Access additional options or context menus |
It’s important to test these interactions rigorously across different devices to ensure compatibility and functionality. For tools that aid in testing and debugging responsive designs, read our article on web development tools.
Understanding and implementing mobile-friendly navigation and touch interactions is key to achieving excellence in responsive web development. Effective execution ensures a seamless and engaging user experience, irrespective of the device they use.
Testing and Debugging
Effective testing and debugging are essential components of responsive web development. Ensuring that a website functions seamlessly across different browsers and devices is critical for delivering a consistent user experience.
Cross-Browser Compatibility
Cross-browser compatibility is a vital aspect of responsive web development. Different browsers interpret HTML, CSS, and JavaScript in diverse ways, which can lead to inconsistencies in how a website is displayed. To achieve cross-browser compatibility, I follow these practices:
- CSS Resets: Implementing CSS resets to standardize the appearance of elements across different browsers.
- Progressive Enhancement: Building the core functionality first and then adding advanced features that enhance the user experience on more capable browsers.
- Vendor Prefixes: Utilizing vendor prefixes for CSS properties to ensure support across various browsers.
Here’s a table to illustrate common vendor prefixes:
Browser | Prefix |
---|---|
Chrome | -webkit- |
Firefox | -moz- |
Internet Explorer | -ms- |
Opera | -o- |
By focusing on these practices, I can create web pages that operate smoothly regardless of the browser being used. For more information on this topic, visit our article on front end web development.
Tools for Responsive Testing
Testing tools are indispensable when developing a responsive website. These tools help me identify and resolve issues related to layout, performance, and cross-browser compatibility.
Some essential tools for responsive testing include:
- Browser Developer Tools: Most modern browsers come with built-in developer tools that facilitate testing and debugging. These tools allow me to simulate different devices and screen sizes, inspect and modify HTML and CSS in real-time, and debug JavaScript.
- Online Testing Services: There are numerous online services that enable me to test how a website looks and performs on various devices and browsers without needing to own the physical hardware.
- Emulators and Simulators: Emulators can replicate the behavior of a specific device or operating system, while simulators mimic the device’s interface and functionalities.
Here’s a table summarizing these tools:
Tool Type | Description |
---|---|
Browser Developer Tools | Built-in tools for inspecting web pages |
Online Testing Services | Platforms for testing on multiple devices |
Emulators | Software to replicate device behavior |
Simulators | Software to mimic device interface |
For a more comprehensive overview of the tools often used in web development, check out our article on web development tools.
Utilizing these tools and techniques ensures that I can deliver a responsive, functional website that provides an optimal user experience across various devices and browsers. More insights into best practices can be found in our section on full stack web development.