Reading time: 2 minutes.
PHP, a widely-used scripting language, offers various built-in functions for manipulating strings, one of which is the PHP explode()
function. This function is essential for developers working with string data, as it allows for splitting a string into an array based on a delimiter. Understanding how to use the explode()
function effectively can significantly enhance data handling in PHP.
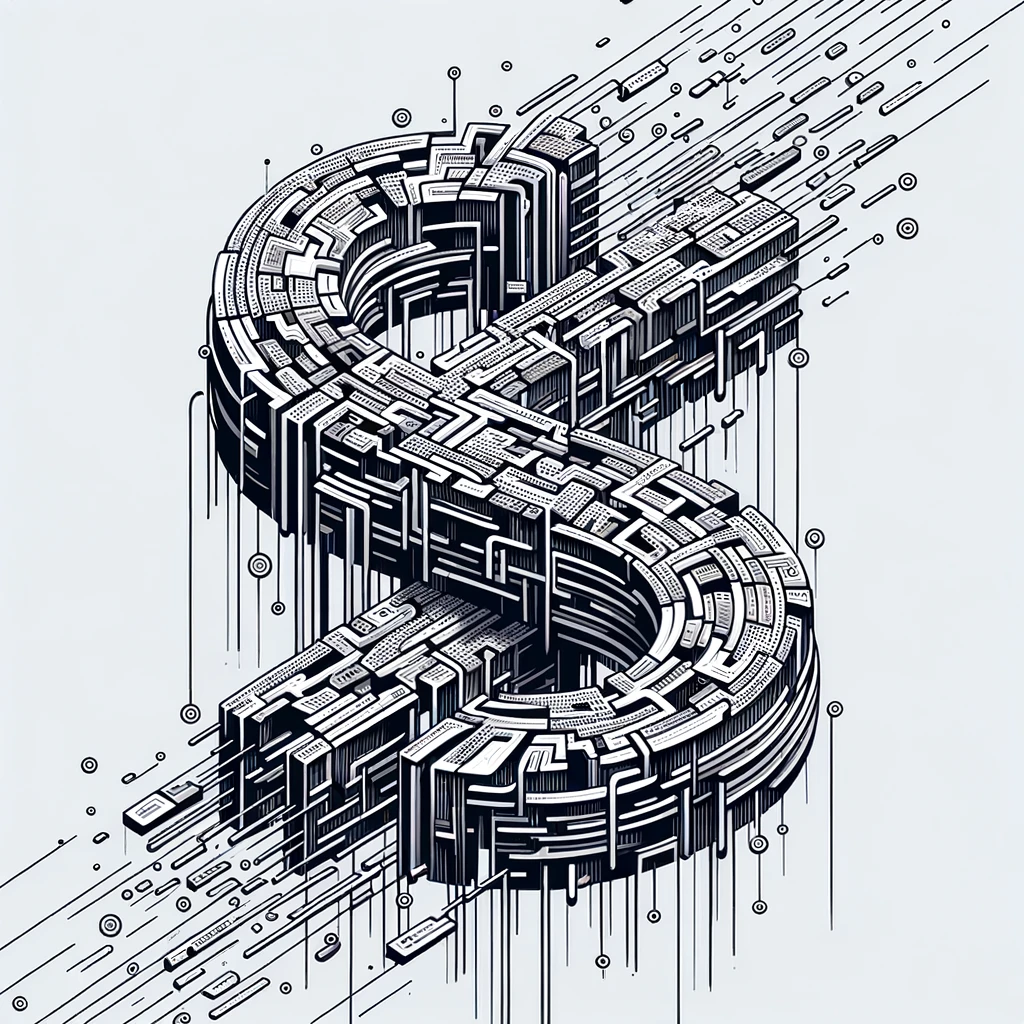
What is the PHP Explode Function?
The explode()
function in PHP splits a string into an array by a specified delimiter. The syntax of the function is:
array explode ( string $delimiter , string $string [, int $limit ] )
$delimiter
: This parameter specifies the boundary string, which is used to break the$string
into parts.$string
: The input string to be split.$limit
: An optional parameter that determines the maximum number of elements in the returned array.
Basic Usage of PHP Explode
The basic use of the explode()
function is to split a string into an array. For instance, to split a list of fruits separated by commas:
$fruits = "apple,banana,orange";
$fruitArray = explode(",", $fruits);
The $fruitArray
will contain ['apple', 'banana', 'orange']
.
Understanding the Limit Parameter
The $limit
parameter controls the number of elements in the returned array. The behavior of explode()
changes depending on the value of $limit
:
- If
$limit
is positive, the returned array will contain a maximum of$limit
elements. - If
$limit
is negative, all elements except for the last- $limit
elements are returned. - If
$limit
is zero, the limit is treated as 1.
Example:
$data = "one,two,three,four";
print_r(explode(",", $data, 2)); // Array ( [0] => one [1] => two,three,four )
Handling Empty Strings
When the explode()
function encounters an empty string, it behaves differently based on the delimiter:
- If the delimiter is an empty string,
explode()
will returnFALSE
. - If the string to be exploded is empty,
explode()
will return an array containing one empty string.
Example:
var_dump(explode('', 'test')); // bool(false)
var_dump(explode(',', '')); // array(1) { [0]=> string(0) "" }
Advanced Use Cases
Exploding a String into Key-Value Pairs
explode()
can be used to parse strings into associative arrays. Consider a string representing key-value pairs:
$string = "id:1,name:John Doe,age:30";
$pairs = explode(',', $string);
$result = [];
foreach ($pairs as $pair) {
list($key, $value) = explode(':', $pair);
$result[$key] = $value;
}
print_r($result); // Array ( [id] => 1 [name] => John Doe [age] => 30 )
Nested PHP Explode
For complex strings, nested explode()
calls can be useful. For example, parsing a string representing a matrix:
$matrix = "1,2,3;4,5,6;7,8,9";
$rows = explode(';', $matrix);
$matrixArray = [];
foreach ($rows as $row) {
$matrixArray[] = explode(',', $row);
}
print_r($matrixArray);
// Array ( [0] => Array ( [0] => 1 [1] => 2 [2] => 3 )
// [1] => Array ( [0] => 4 [1] => 5 [2] => 6 )
// [2] => Array ( [0] => 7 [1] => 8 [2] => 9 ) )
Common Mistakes and Tips
- Choosing the Right Delimiter: Ensure the delimiter accurately represents the boundary in your string.
- Handling Delimiters in Data: If your data might contain the delimiter, consider using a more unique delimiter or escape characters.
- Type Casting: Remember that
explode()
returns an array of strings. Type cast as needed.
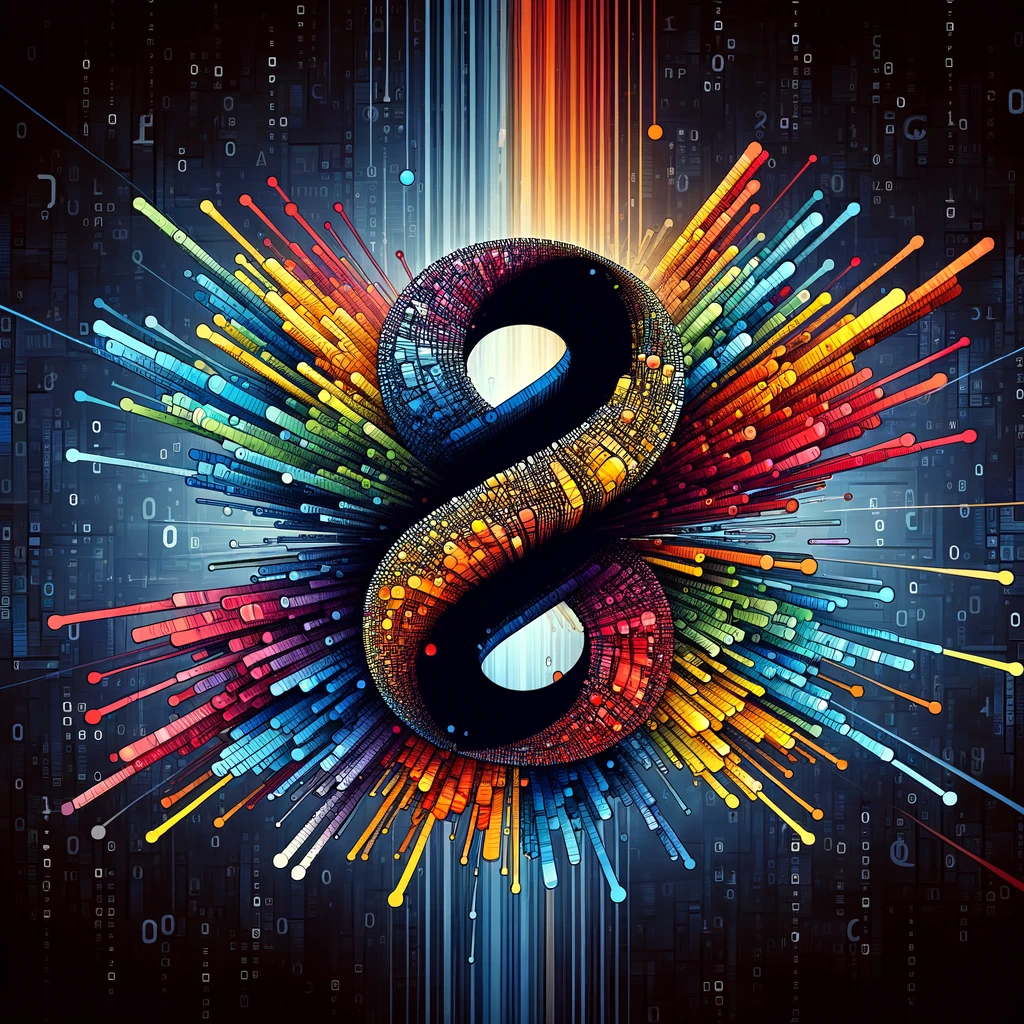
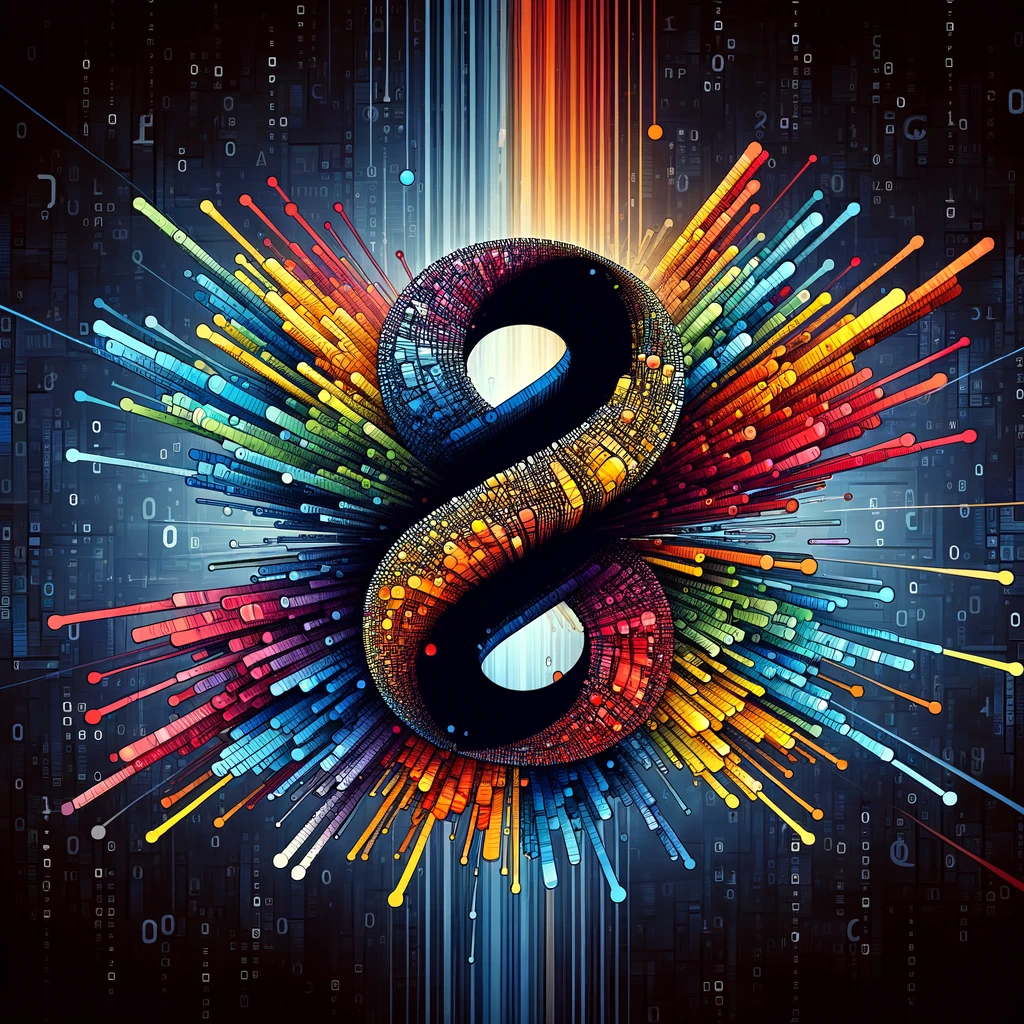
Conclusion
The explode()
function is a powerful tool in PHP for handling string data. Whether you’re dealing with simple lists or complex data structures, mastering explode()
will improve your data manipulation capabilities. Remember to consider edge cases and the structure of your data to make the best use of this function.
This guide provides a comprehensive overview of the explode()
function in PHP, covering its syntax, basic and advanced use cases, and some common mistakes and tips. It’s designed to give