Reading time: 8 minutes.
In the ever-evolving world of web development, staying on top of the latest tools and technologies is crucial. Among these innovations, AI has been making significant strides, particularly in the realm of natural language processing. One such marvel is OpenAI’s ChatGPT, a model known for its conversational prowess. But how does it fare in more technical tasks, like creating CSS? Intrigued, I decided to put ChatGPT to the test.
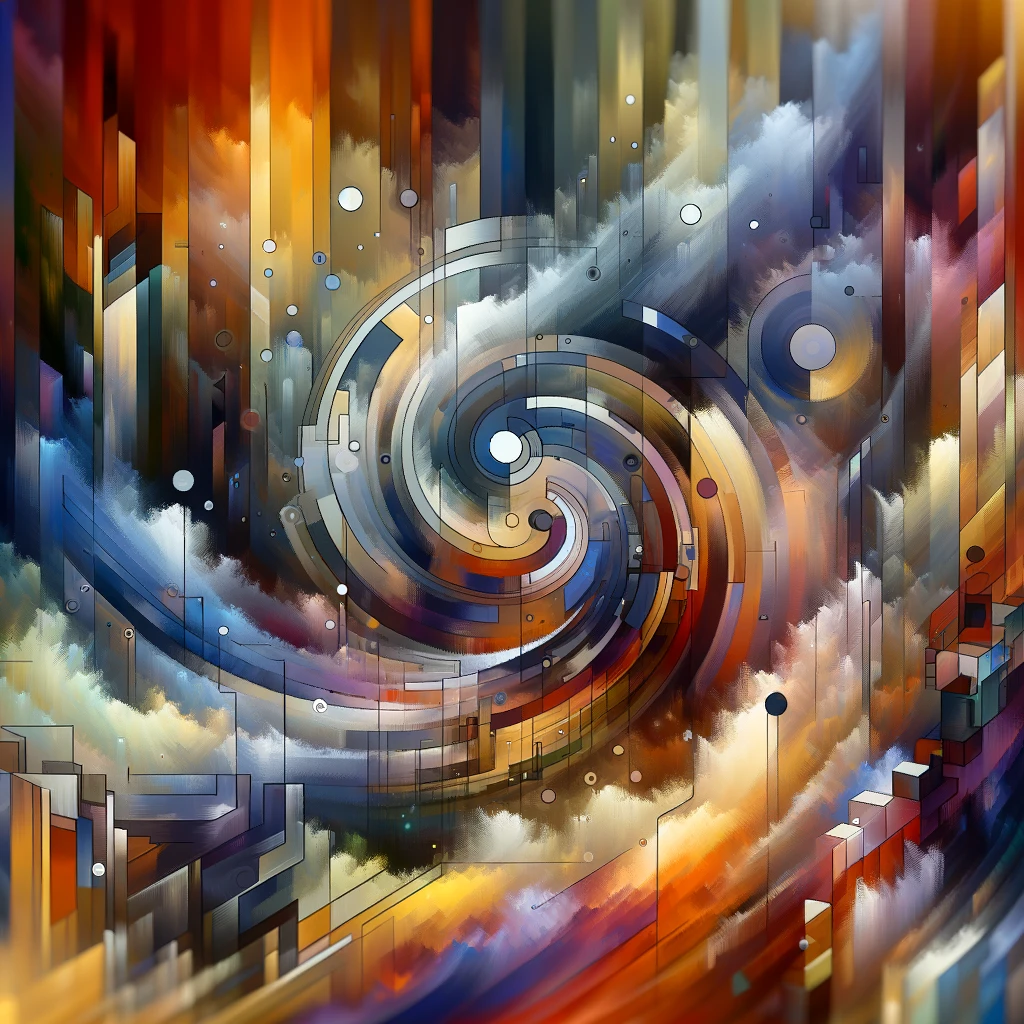
Initial Impressions
At first glance, ChatGPT impresses with its ability to understand and respond to a wide range of queries. Its natural language processing capabilities are top-notch, making it an ideal candidate for a variety of tasks. However, creating CSS is a different ball game. It’s not just about understanding language, but also about translating requirements into functional and aesthetic code.
The Experiment
To test ChatGPT’s CSS capabilities, I set up a series of tasks, each increasing in complexity. The goal was to evaluate not just the accuracy of the code but also its efficiency and modernity.
Task 1: Basic Styling
The first task was straightforward – create a simple CSS snippet to style a header. I asked ChatGPT to provide CSS code for a header with specific font, color, and size. The result was surprisingly accurate. The code was not only correct but also followed best practices, like using em
units for font size and rgba
for color, offering flexibility and better scaling.
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple Styled Header</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<header>
<h1>My Awesome Website</h1>
</header>
</body>
</html>
CSS Code
/* styles.css */
header {
background-color: rgba(100, 100, 250, 0.5); /* Light blue background with some transparency */
padding: 20px;
text-align: center;
}
header h1 {
font-family: 'Arial', sans-serif; /* Arial font */
font-size: 2em; /* 2 times the default size */
color: white; /* White text color */
margin: 0; /* Remove default margin */
}
In this example, the HTML structure includes a <header>
tag containing an <h1>
element. The CSS file (styles.css
) styles the <header>
with a semi-transparent light blue background, center alignment, and padding. The <h1>
element inside the header is styled with the Arial font, set to twice the default size, and colored white. This creates a simple yet visually appealing header for a website.
Task 2: Responsive Design
Next, I challenged ChatGPT to create a CSS snippet for a responsive navigation bar. This task required a deeper understanding of CSS principles, especially media queries. ChatGPT’s response was on point, demonstrating an understanding of responsive design. The code included a media query for smaller screens and used relative units for scalability.
HTML Snippet for Responsive Navigation Bar
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Navigation Bar</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<nav class="navbar">
<ul class="nav-list">
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Services</a></li>
<li><a href="#">Contact</a></li>
</ul>
</nav>
</body>
</html>
CSS Snippet for Responsive Navigation Bar
/* Basic Styling for Navigation Bar */
.navbar {
background-color: #333;
overflow: hidden;
}
.nav-list {
list-style-type: none;
margin: 0;
padding: 0;
display: flex;
justify-content: flex-start;
}
.nav-list li {
float: left;
}
.nav-list li a {
display: block;
color: white;
text-align: center;
padding: 14px 16px;
text-decoration: none;
}
.nav-list li a:hover {
background-color: #ddd;
color: black;
}
/* Responsive Design */
@media screen and (max-width: 600px) {
.nav-list {
flex-direction: column;
width: 100%;
}
.nav-list li {
width: 100%;
}
.nav-list li a {
padding: 10px;
border-bottom: 1px solid #fff;
}
}
In this setup, the navigation bar is styled with basic CSS properties for a horizontal layout. The media query kicks in for screen widths of 600 pixels or less, changing the navigation to a vertical layout, suitable for smaller screens like mobile devices. This demonstrates how to create a basic but functional responsive navigation bar using HTML and CSS.
Task 3: CSS Grid Layout
For a more advanced test, I asked ChatGPT to create a layout using CSS Grid. This required a more complex understanding of CSS layout techniques. ChatGPT’s response was impressive, providing a clean, well-structured grid layout. It used modern CSS properties like grid-template-columns
and grid-gap
, showing an up-to-date knowledge of CSS.
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Grid Layout Example</title>
<link rel="stylesheet" type="text/css" href="styles.css">
</head>
<body>
<div class="grid-container">
<div class="grid-item">Item 1</div>
<div class="grid-item">Item 2</div>
<div class="grid-item">Item 3</div>
<div class="grid-item">Item 4</div>
<div class="grid-item">Item 5</div>
<div class="grid-item">Item 6</div>
</div>
</body>
</html>
This HTML structure sets up a container (grid-container
) with several items (grid-item
) inside it. Each grid-item
represents a cell in the grid.
CSS (styles.css
):
.grid-container {
display: grid;
grid-template-columns: repeat(3, 1fr); /* Creates a 3-column layout */
grid-gap: 10px; /* Spacing between grid items */
padding: 10px;
}
.grid-item {
background-color: #f0f0f0; /* Light grey background for each item */
border: 1px solid #ccc; /* Grey border */
padding: 20px;
text-align: center;
font-size: 16px;
}
/* Responsive design: 2-column grid for smaller screens */
@media (max-width: 600px) {
.grid-container {
grid-template-columns: repeat(2, 1fr);
}
}
This CSS defines a simple grid layout for the .grid-container
with 3 columns. The grid-gap
property adds spacing between the items. The grid-template-columns
property is set to repeat(3, 1fr)
, which creates three columns of equal width.
Additionally, a media query is included for responsive design. When the screen width is 600 pixels or less, it changes the grid to a 2-column layout.
This code snippet demonstrates how ChatGPT can generate a functional and modern grid layout using CSS Grid, which is responsive and adaptable to different screen sizes.
Task 4: Animation with Keyframes
The final challenge was to create a simple animation using keyframes. This task was the most complex, requiring an understanding of CSS animations and syntax. ChatGPT managed to produce a basic animation, though it lacked some refinement compared to what a seasoned web developer might write. However, it was functional and correctly utilized the @keyframes
rule.
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple CSS Animation</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<button class="animated-button">Hover Over Me!</button>
</body>
</html>
This HTML sets up a basic page with a button element. The button has a class animated-button
which we will target in our CSS.
CSS Code
.animated-button {
background-color: #4CAF50;
color: white;
padding: 15px 32px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 4px 2px;
cursor: pointer;
border: none;
transition: background-color 0.3s ease;
}
.animated-button:hover {
animation: pulse 1s infinite;
}
@keyframes pulse {
0% {
transform: scale(1);
opacity: 1;
}
50% {
transform: scale(1.1);
opacity: 0.7;
}
100% {
transform: scale(1);
opacity: 1;
}
}
In this CSS snippet, the .animated-button
class styles the button. When the button is hovered over, it triggers an animation named pulse
. The @keyframes
rule defines this pulse
animation, which scales the button up to 1.1 times its original size and changes its opacity at the midpoint, creating a pulsing effect.
This is a basic example of CSS animation using keyframes. You can modify the keyframes and other properties like animation-duration
, animation-timing-function
, etc., to create various animation effects.
Analysis
After reviewing the results, a few key observations stood out:
- Accuracy: ChatGPT demonstrated a high level of accuracy in translating requirements into CSS code. Even complex tasks like grid layout and basic animations were handled well.
- Best Practices: The code snippets followed modern best practices, using relative units and modern CSS properties.
- Limitations: While ChatGPT excelled in basic to moderately complex tasks, its output for highly complex or nuanced CSS tasks might require further refinement from a human developer.
Implications for Web Development
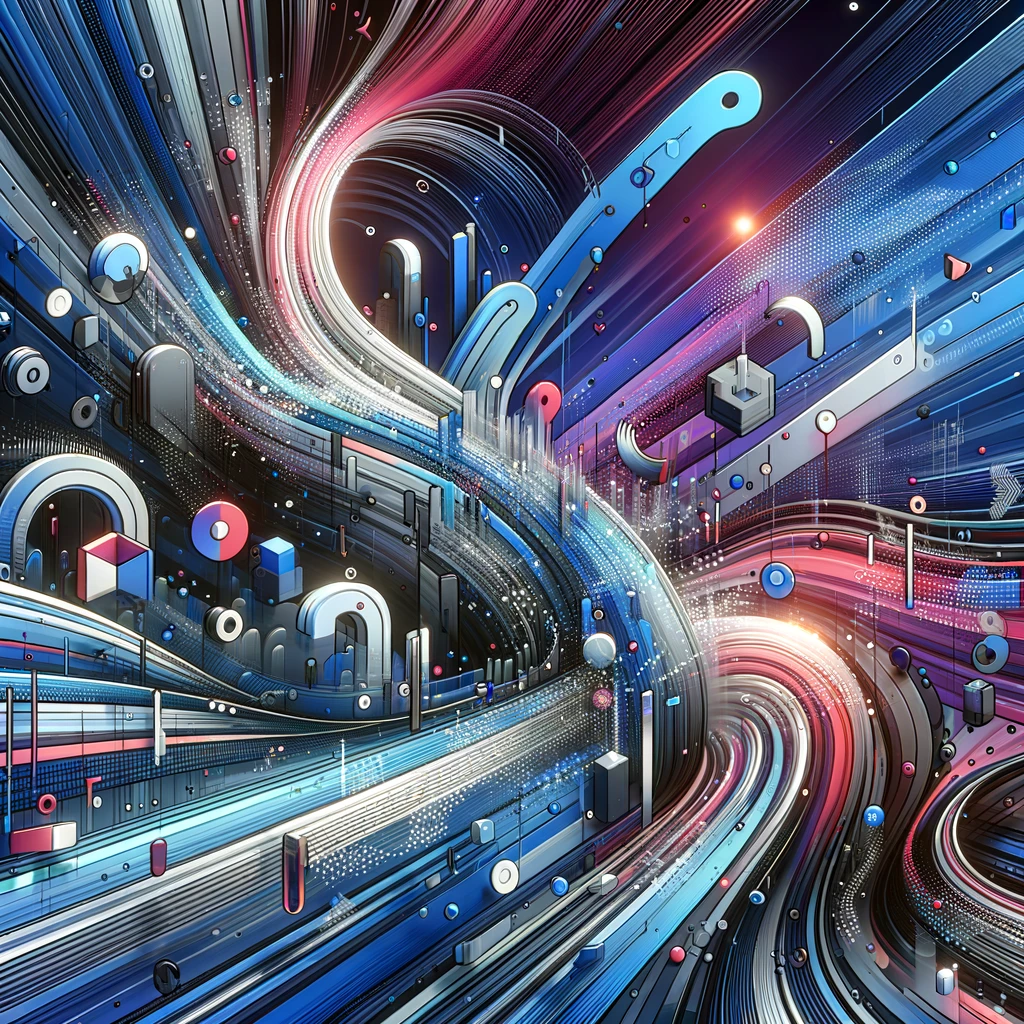
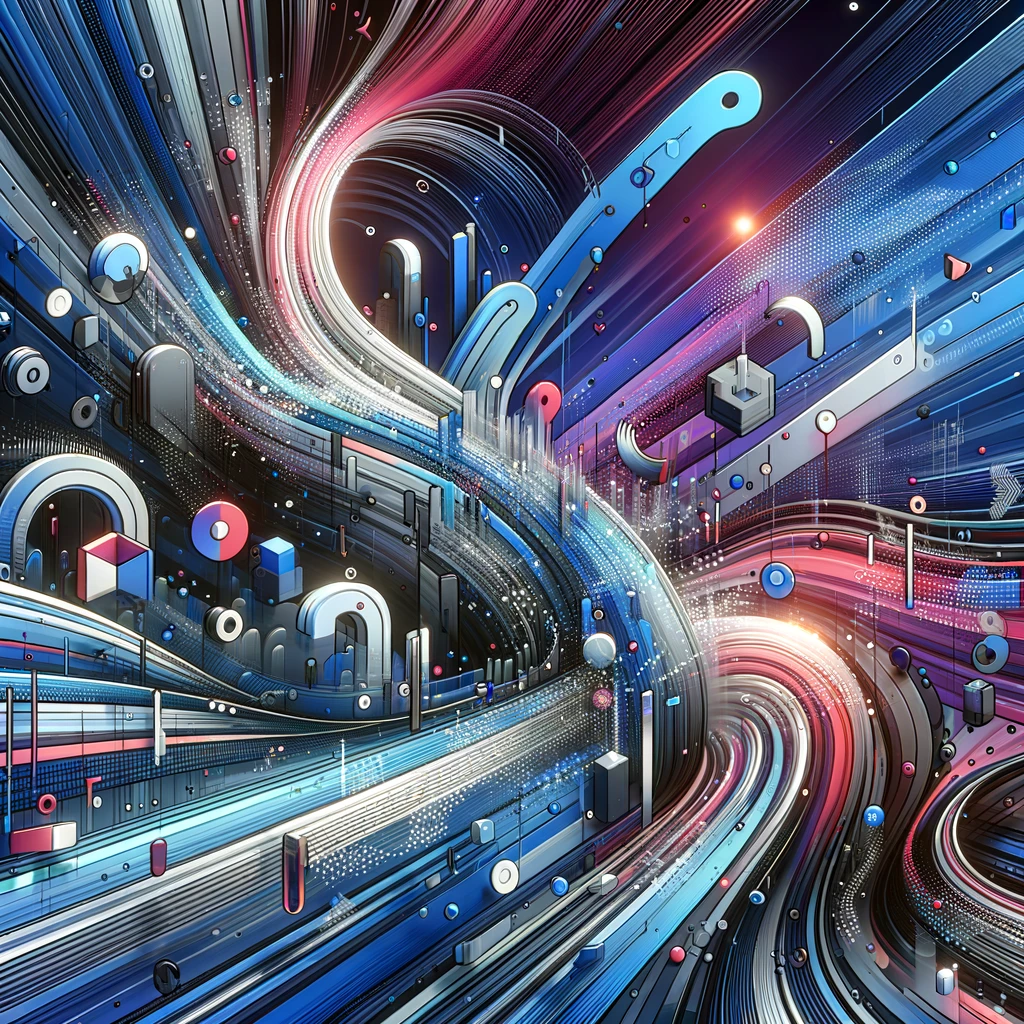
The implications of these findings are significant for the field of web development. ChatGPT could serve as an assistant, helping with quick CSS solutions or offering syntax reminders. It could be particularly useful for beginners learning CSS or for developers looking to speed up their workflow.
Ethical and Practical Considerations
While ChatGPT’s potential in web development is promising, it’s essential to consider the ethical and practical aspects. Relying too heavily on AI for code generation might lead to a lack of understanding of underlying principles among new developers. Moreover, while ChatGPT can generate code, it doesn’t yet have the capability to understand the nuances of visual design and user experience, which are critical in web development.
Conclusion
In conclusion, my experiment with ChatGPT and CSS was a success. ChatGPT proved capable of generating functional and modern CSS code, handling a range of tasks from basic styling to more complex layouts and animations. While it’s not a replacement for human expertise, especially in more nuanced or complex scenarios, it’s an incredibly useful tool that can augment the capabilities of web developers at all levels.
As AI continues to evolve, its role in web development and other technical fields is likely to grow. For now, ChatGPT stands as a testament to the incredible potential of AI in the realm of coding and beyond.
Pingback: The Future of Work: Adapting to a Rapidly Evolving Job Landscape