Reading time: 3 minutes.
PHP, a widely used scripting language for web development, offers a rich set of functions for string manipulation. Among them, PHP strpos
is a fundamental function that developers often use. This post will guide you through the essentials of using strpos
in PHP, covering its purpose, syntax, usage, common pitfalls, and best practices.
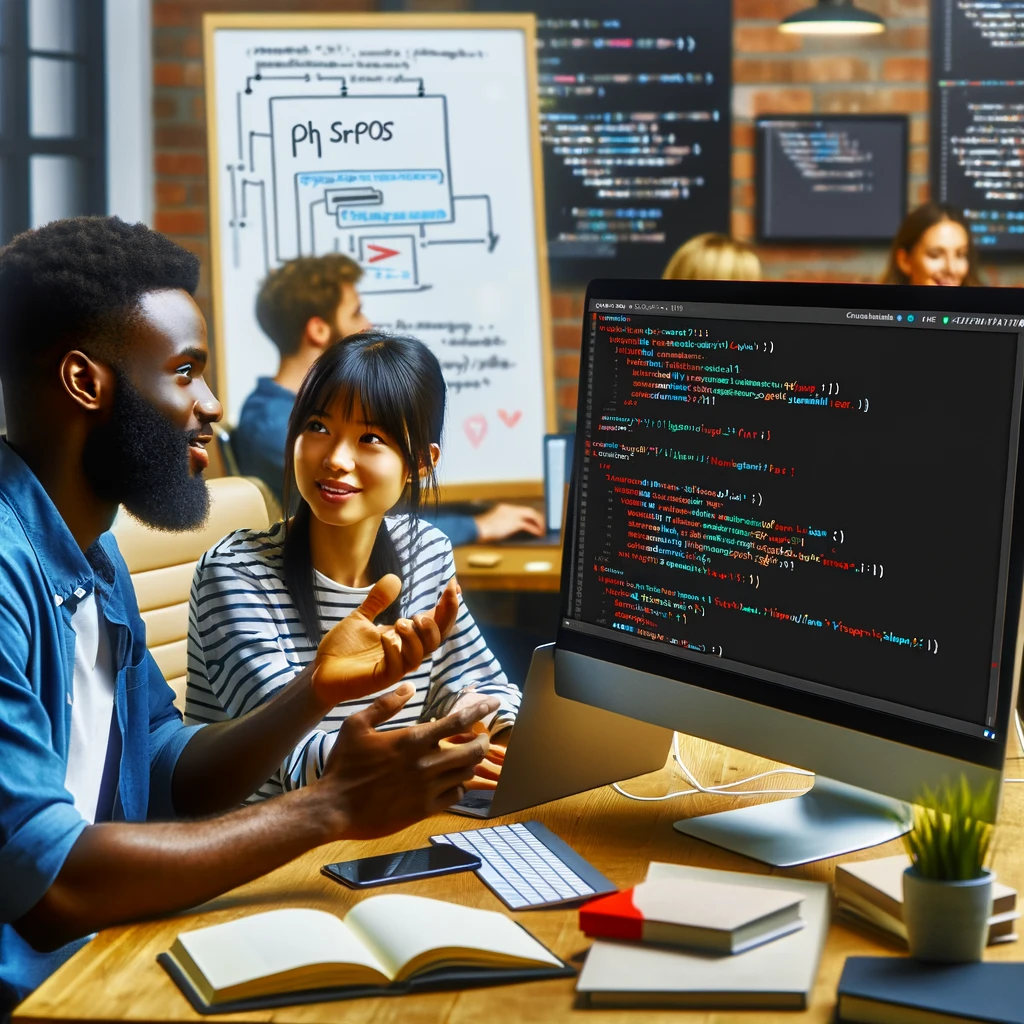
What is PHP strpos?
strpos
, short for “string position,” is a built-in PHP function that finds the position of the first occurrence of a substring in a string. It’s particularly useful when you want to determine if a certain piece of text exists within a string and where it is located.
Syntax
The basic syntax of PHP strpos
is as follows:
int strpos ( string $haystack , mixed $needle [, int $offset = 0 ] )
$haystack
: The string to search in.$needle
: The substring to search for.$offset
: Optional. Specifies where in$haystack
to start searching. The default is 0, which means it starts at the beginning of the string.
Return Values
strpos
returns the position of the $needle
in the $haystack
as an integer. It’s important to note that string positions in PHP start at 0, not 1. If the substring is not found, strpos
returns false
.
Common Usage of PHP strpos
- Check if a Substring Exists in a String To check if a substring is present in a string, you can use
strpos
in anif
statement:
$myString = "Hello, world!";
$findMe = "world";
if (strpos($myString, $findMe) !== false) {
echo "'$findMe' found in string";
} else {
echo "'$findMe' not found in string";
}
- Case Sensitivity
strpos
is case-sensitive. To perform a case-insensitive search, usestripos
. - Using with an Offset You can specify an offset to start the search from a particular position in the string:
$myString = "I love PHP. PHP is awesome!";
$findMe = "PHP";
$pos = strpos($myString, $findMe, 10);
if ($pos !== false) {
echo "'$findMe' found in the string at position $pos";
} else {
echo "'$findMe' not found in the string";
}
Common Pitfalls of PHP strpos
- Handling
false
and 0 Properly Sincestrpos
returnsfalse
if the substring is not found, and 0 if the substring is at the start of the string, it’s essential to use the!==
operator to avoid confusion betweenfalse
and 0:
if (strpos($myString, $findMe) !== false) {
// Correct way
}
- Incorrect Offset Usage Be careful with the offset parameter. An offset that is greater than the string length will cause
strpos
to returnfalse
.
Best Practices
- Clear Variable Naming Use clear and descriptive names for your variables (
$haystack
and$needle
) to make your code more readable. - Error Handling Always check the return value of
strpos
to handle the case where the substring is not found. - Use
mb_strpos
for Multibyte Strings If you’re dealing with multibyte character encodings, like UTF-8, usemb_strpos
instead ofstrpos
for accurate results. - Performance Consideration
strpos
is a fast and efficient way to find substrings. However, if you’re only checking for the existence of a substring and not its position, usingstrstr
orstr_contains
(as of PHP 8) might be more semantic.
Conclusion
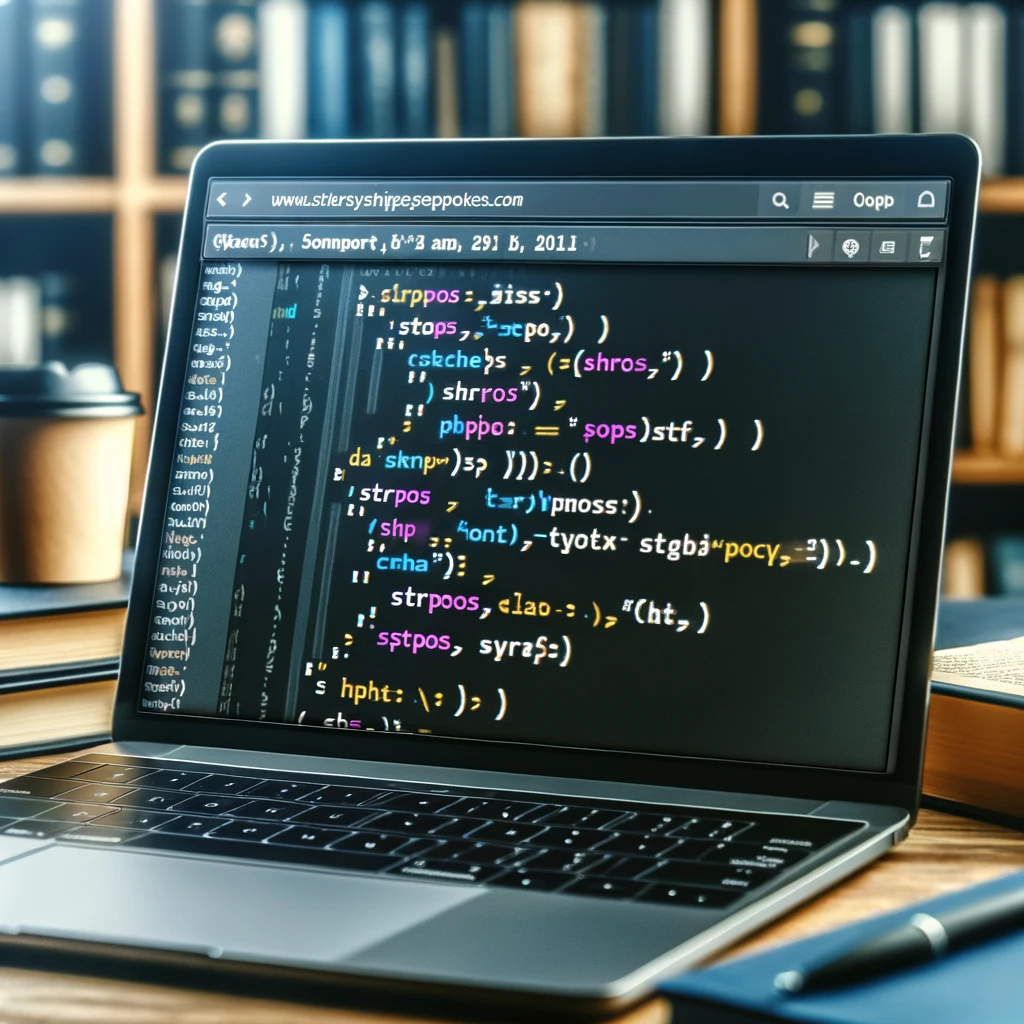
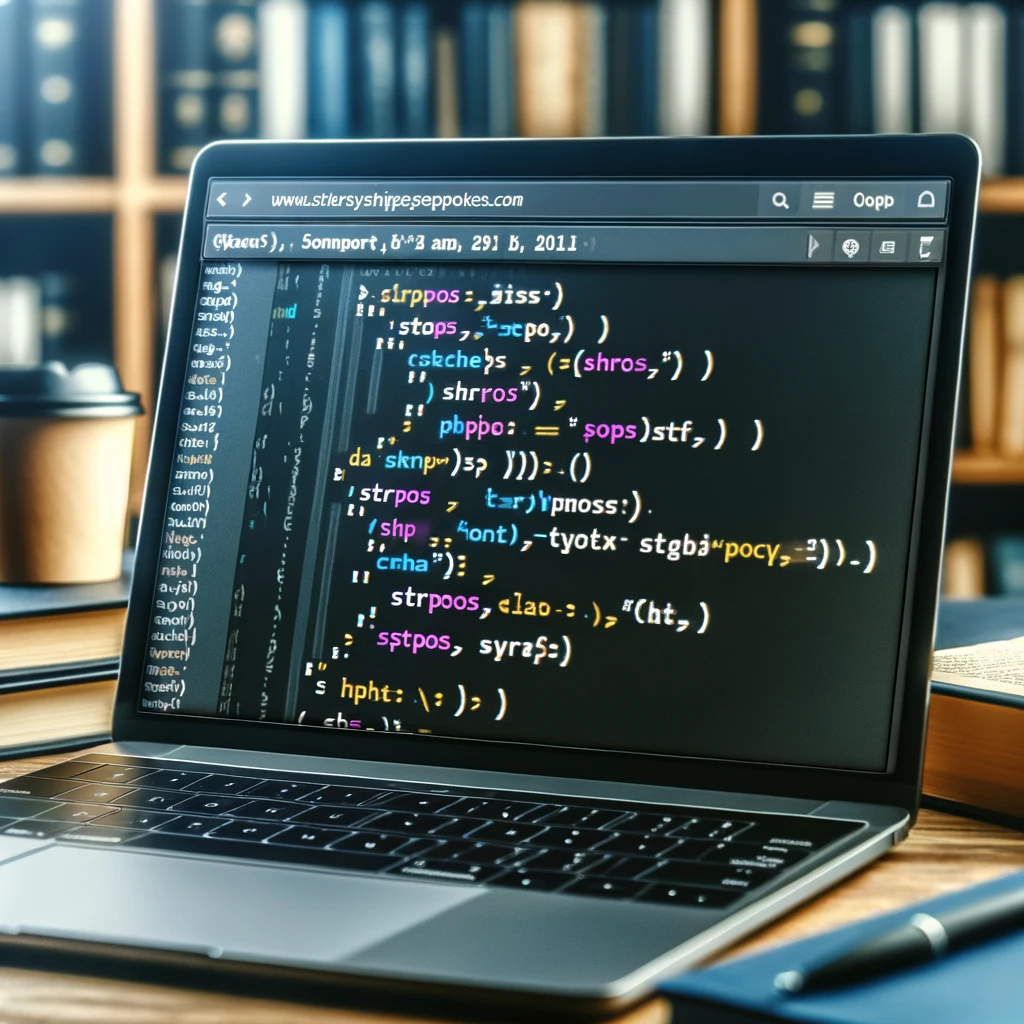
Understanding and utilizing PHP strpos
effectively is crucial for PHP developers dealing with string manipulation. By following the syntax, being aware of common pitfalls, and adhering to best practices, you can use strpos
to its full potential, making your PHP code more robust and efficient.
Remember, the key to mastering any PHP function lies in practice and understanding its nuances. So, experiment with strpos
in different scenarios to deepen your understanding.
This post has walked you through the essentials of using PHP strpos
. Whether you’re a beginner or an experienced developer, mastering such fundamental functions is pivotal in your journey through PHP development. Keep coding, and keep exploring!