Reading time: 3 minutes.
When working with MySQL, a common requirement is to obtain the mysql row number for each record in a result set. However, unlike some other SQL databases, MySQL does not provide a built-in row number function. This means that users must find alternative methods to achieve this functionality. In this post, we will explore how to generate row numbers in a MySQL query using a simple yet effective method.
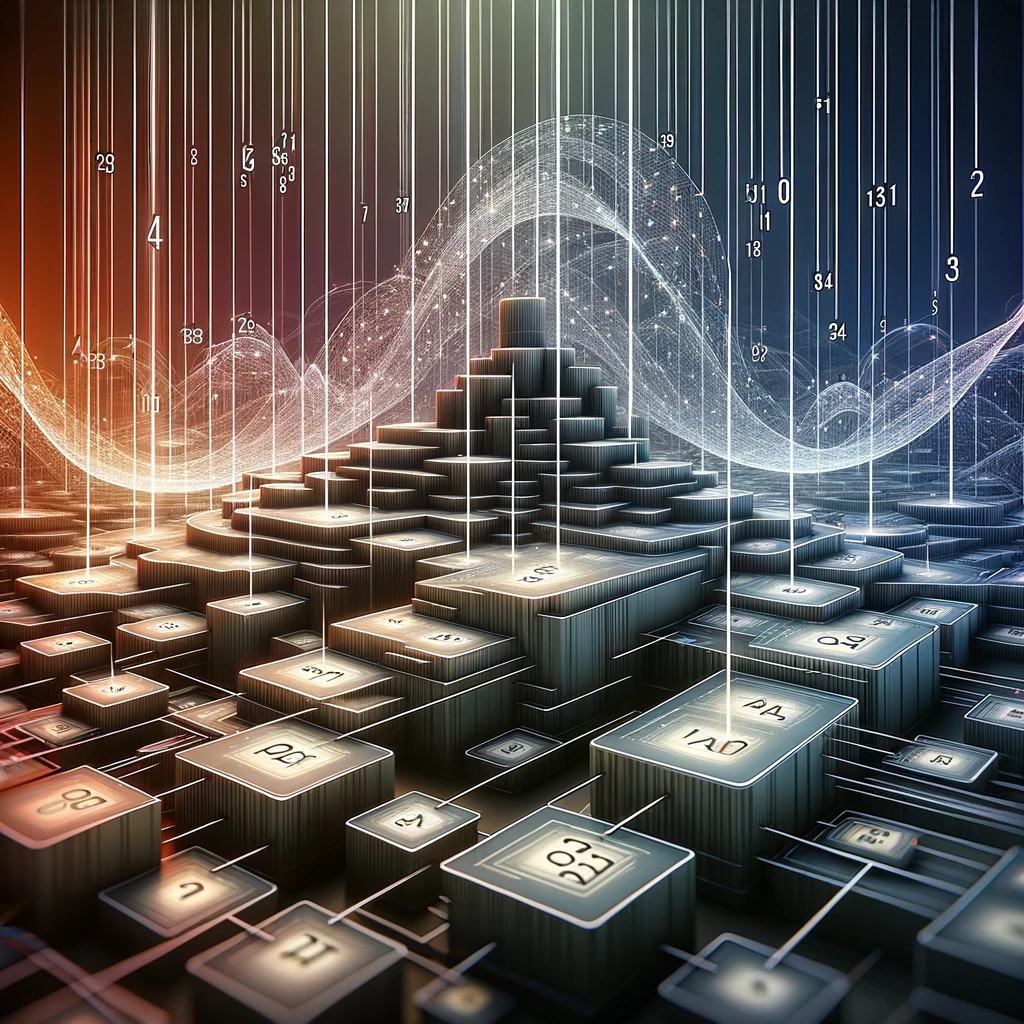
Understanding the Challenge of MySQL row number
In SQL databases, the row number of a record typically refers to its position within a set of rows returned by a query. This can be particularly useful for tasks like pagination or ranking results. While databases like SQL Server and Oracle provide built-in functions (ROW_NUMBER()
for example), MySQL requires a different approach.
The MySQL row number Solution
To simulate the MySQL row number functionality, you can use session variables. These are variables that are specific to your current connection to the database. You can increment these variables within your query to mimic the behavior of a row number. Here’s a basic template for how this can be done:
SELECT mt.*,
@rownum := @rownum + 1 AS row_number
FROM MY_TABLE mt,
(SELECT @rownum := 0) r
In this query, YOUR_TABLE
should be replaced with the name of your actual table. Let’s break down what this query does:
- Select from Your Table: The
FROM YOUR_TABLE t
part of the query is where you specify the table from which to retrieve the records. - Initializing the Row Number: The
(SELECT @rownum := 0) r
is a subquery that initializes a session variable named@rownum
to 0. This subquery is joined with the main table (YOUR_TABLE
). - Incrementing the Row Number: Within the
SELECT
statement,@rownum := @rownum + 1
increments the@rownum
variable for each row processed in the query. The result is aliased asrank
, which effectively acts as the row number.
Practical Example of MySQL Row Number
Let’s consider a practical example. Suppose you have a table named employees
and you want to retrieve all employees with their row numbers. Your query would look something like this:
SELECT e.*,
@rownum := @rownum + 1 AS row_number
FROM employees e,
(SELECT @rownum := 0) r
This query will return all columns from the employees
table, along with a row_number
column that indicates the row number of each employee.
Things to Keep in Mind
While this method is effective, there are a few things you should keep in mind:
- Session Variables: Since this solution uses session variables, the value of
@rownum
persists only during the session. It gets reset when the session ends. - Order of Results: The row number assignment depends on the order in which MySQL processes the rows. If you need the row numbers in a specific order, you should use an
ORDER BY
clause. - Performance: For large datasets, this method might affect performance. It’s always a good idea to test the query with your dataset to ensure it meets your performance expectations.
- MySQL Versions: Be aware of the MySQL version you are using. The behavior of session variables can vary between versions.
Conclusion
In summary, while MySQL does not provide a built-in row number function, you can effectively simulate this functionality using session variables. This method is straightforward and versatile, allowing you to add row numbers to your queries without much hassle. Always remember to consider the order and performance implications when using this technique.
By understanding and utilizing these concepts, you can enhance your MySQL queries to include row numbers, thereby expanding the range of data operations you can perform. This is just one example of how thinking creatively with SQL can help you overcome the limitations of specific database systems.