Reading time: 6 minutes.
PHP and Python are two of the most popular programming languages used today, each with its unique characteristics and uses. They serve different purposes but are often compared due to their widespread use in web development. This article explores the main differences between PHP and Python, covering aspects from their syntax and performance to their community support and typical applications.
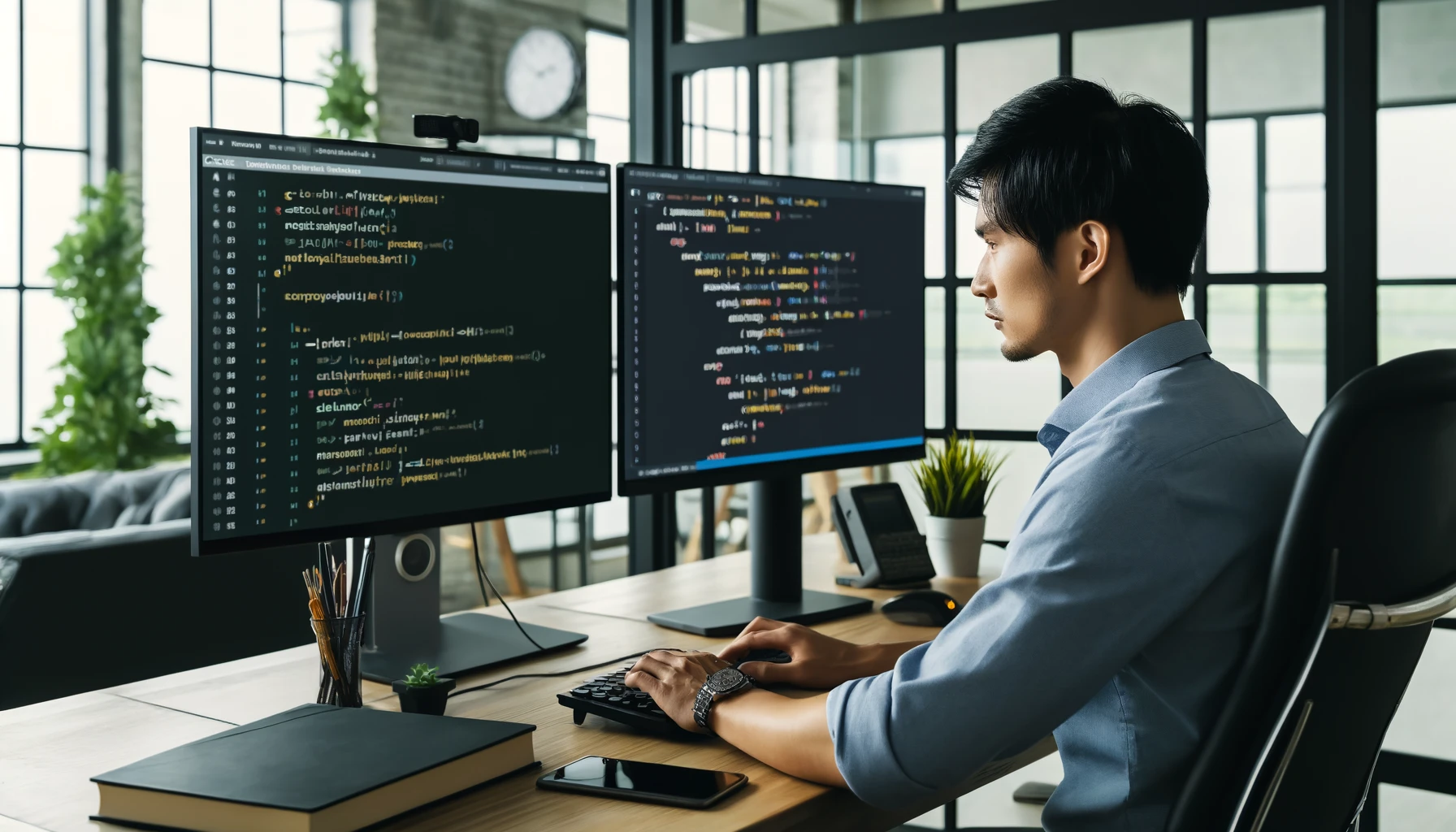
Historical Background
PHP
PHP (Hypertext Preprocessor) was created in 1994 by Rasmus Lerdorf. It was initially designed as a set of Common Gateway Interface (CGI) binaries written in the C programming language. Over the years, PHP has evolved significantly and is primarily used for server-side scripting and web development. PHP is the backbone of popular content management systems (CMS) like WordPress, Joomla, and Drupal.
Python
Python was created earlier than PHP, in 1991, by Guido van Rossum. It is a general-purpose language known for its readability and straightforward syntax. Python’s design philosophy emphasizes code readability and simplicity, which reduces the cost of program maintenance. Python supports multiple programming paradigms, including procedural, object-oriented, and functional programming. It is widely used in web development, data analysis, artificial intelligence (AI), scientific computing, and more.
Syntax and Ease of Learning
PHP
PHP’s syntax borrows heavily from C and Perl, offering a style that is familiar to those with experience in these languages. This familiarity can be a significant advantage, easing the transition for developers proficient in these traditional, structured programming languages. In PHP, scripts are commonly embedded directly within HTML code, making it particularly suited for rapidly creating dynamic web pages. This integration allows developers to quickly mix PHP logic with HTML layout without the need for additional templating systems. However, this blending of code can sometimes lead to cluttered and difficult-to-maintain codebases, particularly in large projects where clean architecture is critical.
Moreover, PHP’s flexibility with variable usage—such as not requiring explicit declaration and allowing dynamic typing—can make it easier for beginners to start coding. However, this same flexibility can lead to bugs and security issues if variables are not handled carefully. PHP also offers extensive documentation and a large array of user-contributed notes and comments, which can be immensely helpful for beginners trying to understand the nuances of the language.
Python
Python is renowned for its straightforward and readable syntax, which is often described as nearly pseudocode, due to its clean and expressive style. This simplicity in syntax makes Python exceptionally easy for beginners to learn and understand. Python enforces indentation as part of its syntax, ensuring that code readability is a requirement, not an option. This has made Python a preferred language in educational environments, where learning programming fundamentals without getting overwhelmed by complex syntax is crucial.
Python’s syntax promotes good programming practices by design. The language’s structure encourages developers to produce clean, readable code that is easy to maintain and scale. This characteristic, combined with its powerful standard libraries and the supportive Python community, allows beginners to progress quickly in learning the language and to start working on real-world projects in a relatively short amount of time. Furthermore, Python’s extensive use in diverse fields—from web development to artificial intelligence—provides learners with a vast landscape of opportunities to apply their skills in practical, impactful ways.
Performance
PHP
PHP has improved significantly in speed with its latest versions, particularly PHP 7.x, which has drastically optimized performance and reduced memory consumption. However, PHP is typically not as fast as some of the newer technologies like Node.js in handling asynchronous tasks.
Python
Python is not known for its speed but rather for its flexibility and the breadth of its application areas. The language can be slow because it is interpreted and dynamically typed. However, the vast array of libraries and frameworks like NumPy and PyPy can mitigate performance issues, enabling Python to be used effectively for everything from web applications to machine learning tasks.
Libraries and Frameworks
PHP
PHP has a strong collection of frameworks that can accelerate the development of web applications. Some of the most notable frameworks include Laravel, Symfony, and CodeIgniter. These frameworks provide robust tools and features for developing high-quality web applications efficiently.
Python
Python’s ecosystem is one of its greatest strengths, with a comprehensive standard library and a vast selection of third-party modules available for nearly every task imaginable. Frameworks like Django and Flask are particularly popular for web development, while TensorFlow and Scikit-learn are commonly used in data science and machine learning fields.
Community and Support
PHP
PHP has a vast, active community with a plethora of resources and documentation available. The PHP community is very active in creating plugins, frameworks, and tools to help developers.
Python
Python’s community is renowned for being friendly and welcoming to newcomers, which is partly why Python has become so popular among novice programmers. The Python Software Foundation provides extensive documentation, tutorials, and guides to support learners at all levels.
Use Cases
PHP
PHP is predominantly a server-side scripting language used in web development. It is ideal for developing dynamic and data-driven websites and applications. PHP works well with a variety of database management systems, and its role in WordPress development makes it indispensable for many web developers.
Python
Python’s use cases are more diversified. It is used in web development with frameworks like Django and Flask. Beyond web development, Python is a leading language in data analysis, machine learning, AI, and scientific computing. Its versatility also extends to scripting, automation, and creating software applications in business, finance, and academic research.
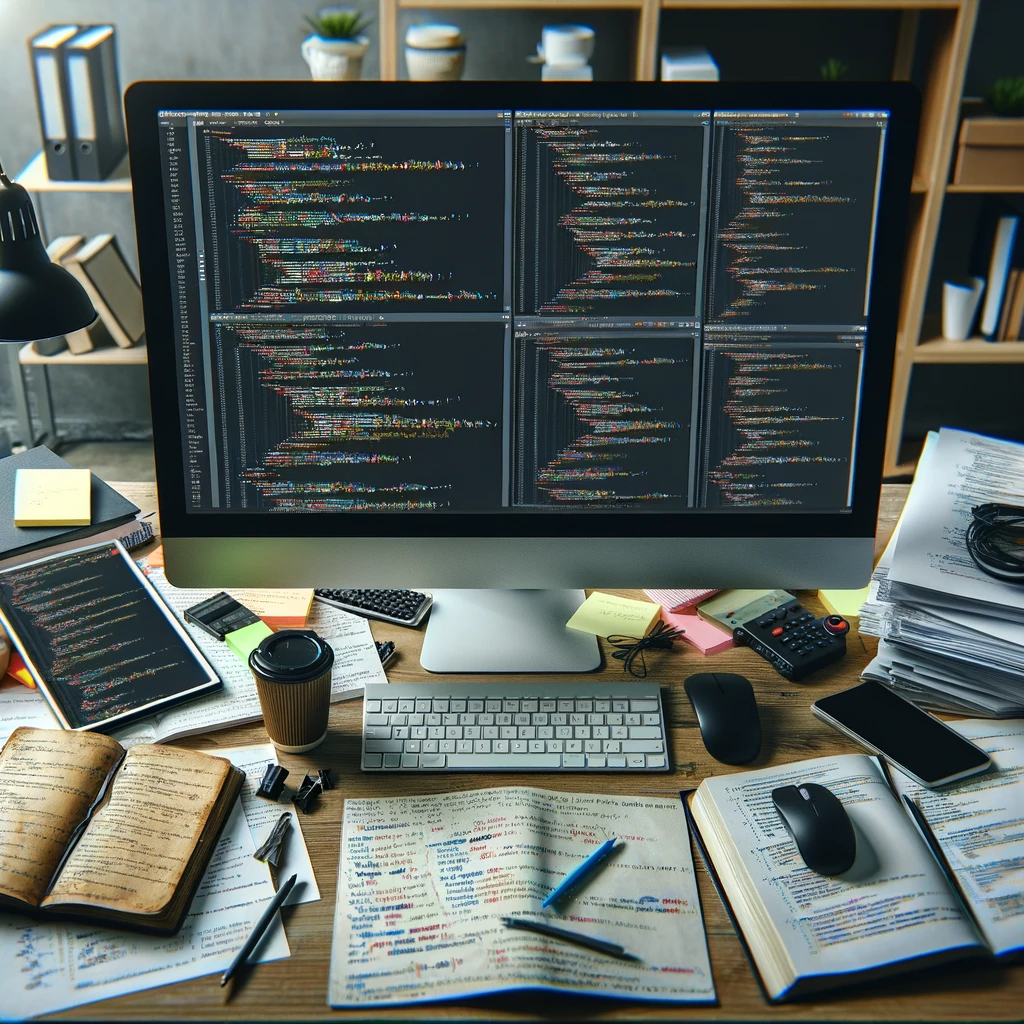
A Simple Example of the Difference (Code)
Sure! Let’s create a simple program in both PHP and Python that checks if a number is prime. A prime number is a number greater than 1 with no divisors other than 1 and itself.
PHP Version
<?php
function isPrime($number) {
if ($number <= 1) {
return false;
}
for ($i = 2; $i <= sqrt($number); $i++) {
if ($number % $i == 0) {
return false;
}
}
return true;
}
// Example usage
$testNumber = 29;
if (isPrime($testNumber)) {
echo "$testNumber is a prime number.";
} else {
echo "$testNumber is not a prime number.";
}
?>
Python Version
def is_prime(number):
if number <= 1:
return False
for i in range(2, int(number**0.5) + 1):
if number % i == 0:
return False
return True
# Example usage
test_number = 29
if is_prime(test_number):
print(f"{test_number} is a prime number.")
else:
print(f"{test_number} is not a prime number.")
Explanation
Both scripts define a function that checks if a number is prime. They follow a similar logic:
- Check if less than or equal to 1: Both functions first check if the number is less than or equal to 1, in which case it cannot be prime, so they return
false
orFalse
. - Loop for divisibility: Both functions then loop from 2 up to the square root of the number (a mathematical optimization to reduce the number of divisions needed). In PHP, this is done using a
for
loop withsqrt($number)
, and in Python withrange(2, int(number**0.5) + 1)
. - Divisibility check: If any divisor is found (i.e., the number is divisible by any number other than 1 and itself without leaving a remainder), the function returns
false
orFalse
. - Return true if prime: If the loop completes without finding a divisor, the function returns
true
orTrue
, indicating the number is prime. - Example usage: Each script tests the function with a number (29 in this case) and prints out whether it is prime or not, demonstrating the function in action.
These examples highlight the syntactical and structural similarities and differences between PHP and Python, while also showcasing each language’s approach to implementing a common algorithmic task.
Conclusion
While both PHP and Python can be used for web development, they differ significantly in design philosophy, ease of use, performance, and application areas. PHP is highly specialized for server-side scripting and web development, while Python is a more versatile language that excels in a wider range of programming tasks from web applications to deep learning.
Choosing between PHP and Python will depend largely on the specific needs of a project, the existing infrastructure, and the developers’ familiarity with the language. Regardless of the choice, both languages offer strong community support, extensive libraries, and continual updates that keep them relevant in the fast-evolving tech landscape.