Reading time: 3 minutes.
JavaScript’s reduce
method is a powerful tool in the arsenal of array methods, often used to derive a single value from an array of values. This versatile function can be employed for a range of tasks, from calculating sums or averages to transforming arrays into more complex data structures like objects or maps. In this article, we will explore the basics of the reduce
method, its syntax, various practical examples, and some advanced use cases to help you fully leverage this function in your JavaScript projects.
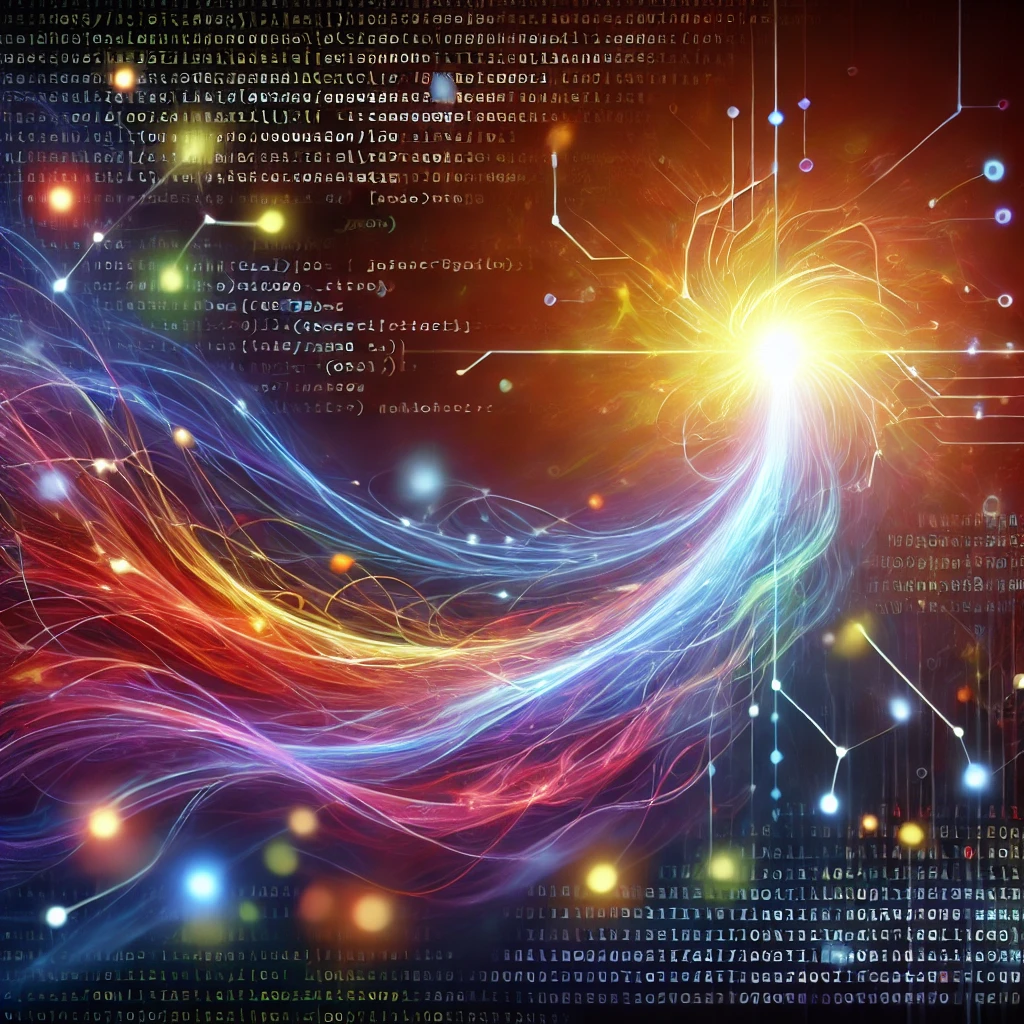
Understanding reduce
The reduce
method in JavaScript is used to execute a reducer function on each element of the array, resulting in a single output value. The reducer function’s return value is accumulated across the elements of the array, which is why it’s often used for operations like summing numbers or concatenating strings.
Syntax of reduce
The reduce
method has the following syntax:
array.reduce(reducerFunction, initialValue)
- reducerFunction: A function that is called on each element of the array, taking four arguments:
accumulator
: Accumulates the callback’s return values; it is the accumulated value previously returned in the last invocation of the callback, orinitialValue
, if supplied.currentValue
: The current element being processed in the array.currentIndex
(optional): The index of the current element being processed in the array.array
(optional): The arrayreduce
was called upon.- initialValue (optional): A value to use as the first argument to the first call of the
reducerFunction
. If no initial value is supplied, the first element in the array will be used as the initialaccumulator
value, and thecurrentValue
will start from the second element.
If the array is empty and no initialValue
is provided, reduce
will throw a TypeError
. If the array only has one element (regardless of position) and no initialValue
is provided, or if initialValue
is provided but the array is empty, the solo value will be returned without calling reducerFunction
.
Basic Example
Let’s start with a simple example to illustrate how reduce
can be used to sum all the numbers in an array:
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((acc, current) => acc + current, 0);
console.log(sum); // Output: 15
In this example, reduce
takes two parameters: a reducer function and an initial value for the accumulator. The reducer function adds the current array item to the accumulator, which starts at zero. After processing all array items, the final value of the accumulator is returned.
Practical Applications of reduce
Calculating Averages
Beyond summing numbers, reduce
can also calculate averages by modifying the reducer function:
const numbers = [10, 20, 30, 40, 50];
const average = numbers.reduce((acc, current, index, array) => {
acc += current;
if (index === array.length - 1) return acc / array.length;
return acc;
}, 0);
console.log(average); // Output: 30
This approach accumulates the total and divides by the array’s length only in the last iteration.
Building Objects
You can also use reduce
to transform an array into an object. For instance, converting an array of properties into a single object:
const keyValuePairs = [['key1', 'value1'], ['key2', 'value2']];
const obj = keyValuePairs.reduce((acc, [key, value]) => {
acc[key] = value;
return acc;
}, {});
console.log(obj); // Output: { key1: 'value1', key2: 'value2' }
Advanced Use Cases
Flattening Arrays
reduce
can flatten nested arrays into a single array:
const nestedArrays = [[1, 2], [3, 4], [5, 6]];
const flatArray = nestedArrays.reduce((acc, current) => acc.concat(current), []);
console.log(flatArray); // Output: [1, 2, 3, 4, 5, 6]
Chaining with Other Array Methods
reduce
can be effectively chained with other array methods like map
and filter
to perform complex transformations and computations:
const transactions = [5.99, 10.49, 9.99, 2.49, 12.99];
const discountThreshold = 10;
const discountedTransactions = transactions
.filter(amount => amount >= discountThreshold)
.map(amount => amount * 0.9)
.reduce((total, amount) => total + amount, 0);
console.log(discountedTransactions.toFixed(2)); // Output: 19.44
Here, filter
removes transactions below $10, map
applies a 10% discount, and reduce
sums up the discounted prices.
Conclusion
The reduce
method is a remarkably flexible tool that, once mastered, can simplify many complex data manipulations in JavaScript. By combining it with other array functions and leveraging it in a variety of scenarios from simple arithmetic to advanced data structures, developers can write more concise and efficient code. Whether you’re summing numbers, computing averages, transforming data structures, or even implementing your custom logic that spans across an array, reduce
provides a robust foundation for iterative solutions and data processing tasks.