Reading time: 3 minutes.
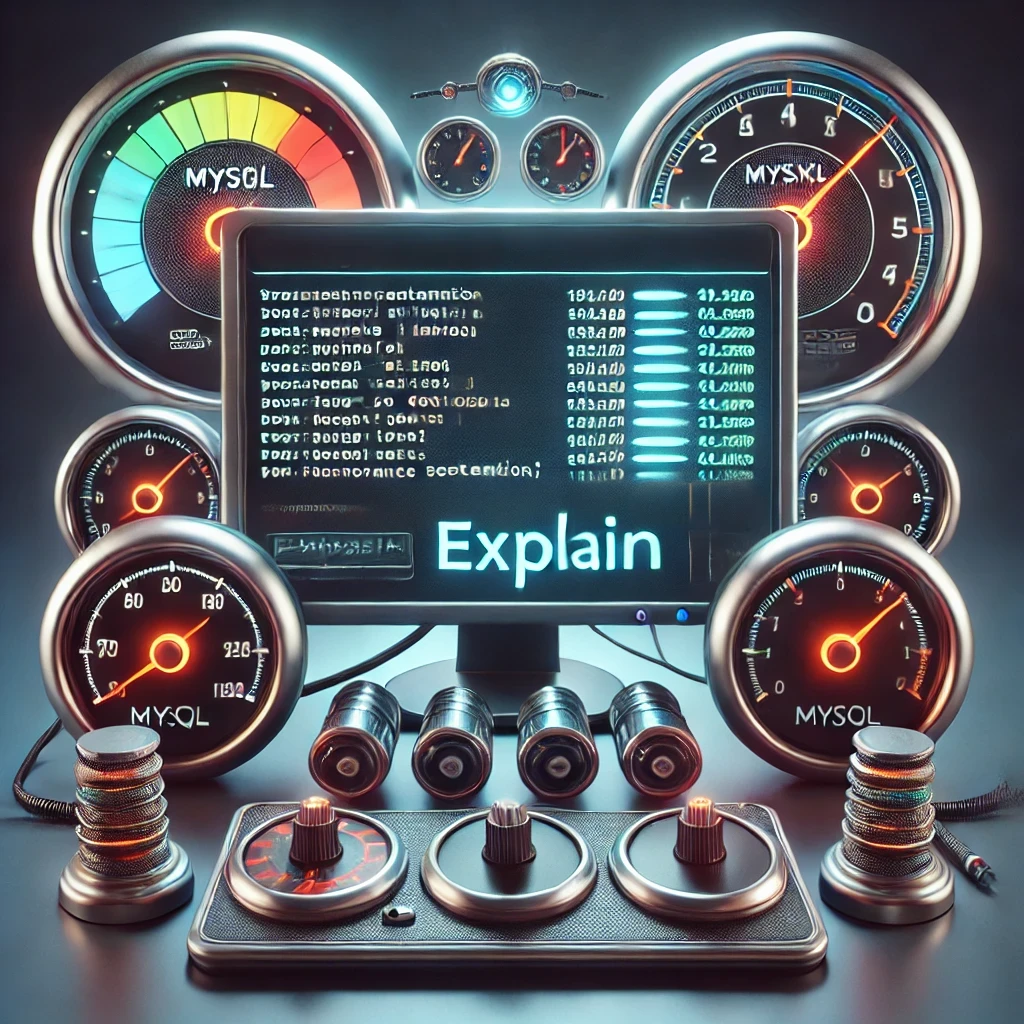
Optimizing queries in MySQL is crucial for ensuring database performance, scalability, and efficiency. Poorly optimized queries can lead to slow response times, high resource consumption, and overall degraded system performance. Here are some best practices and strategies to optimize MySQL queries effectively.
1. Use Indexes Effectively
Indexes significantly speed up data retrieval by reducing the number of rows scanned. The key types of indexes in MySQL include:
- Primary Key Indexes: Automatically created on primary key columns.
- Unique Indexes: Prevent duplicate values and speed up searches.
- Composite Indexes: Useful when querying multiple columns.
- Full-Text Indexes: Optimized for text searching.
Best Practices:
- Index columns that are used in
WHERE
,JOIN
, andORDER BY
clauses. - Avoid over-indexing as it can slow down insert, update, and delete operations.
- Use the
EXPLAIN
command to check if indexes are being used effectively.
2. Use EXPLAIN
to Analyze Query Execution Plans
The EXPLAIN
statement helps understand how MySQL executes queries, allowing for performance tuning.
EXPLAIN SELECT * FROM orders WHERE customer_id = 5;
It provides insights into:
- Table access method (e.g., full scan, index scan, range scan).
- Estimated number of rows examined.
- Use of indexes.
3. Optimize Joins and Subqueries
Joins and subqueries can be expensive in terms of performance. Optimization strategies include:
- Using INNER JOINs over
LEFT JOIN
where possible. - Ensuring both tables have indexed join columns.
- Rewriting subqueries as joins where applicable.
For example, replacing a subquery:
SELECT name FROM customers WHERE id IN (SELECT customer_id FROM orders);
With a JOIN:
SELECT DISTINCT customers.name FROM customers
JOIN orders ON customers.id = orders.customer_id;
This avoids repeated subquery executions and improves performance.
4. Avoid SELECT *
Selecting all columns (SELECT *
) can retrieve unnecessary data, slowing down queries. Instead, specify only the required columns:
SELECT id, name FROM customers WHERE country = 'UK';
This reduces memory and network overhead.
5. Use Proper Data Types and Storage Engines
- Choose appropriate data types (e.g.,
INT
instead ofBIGINT
where possible). - Use
VARCHAR
instead ofTEXT
when indexing is needed. - Select the right storage engine (e.g., InnoDB for transactions and MyISAM for read-heavy operations).
6. Optimize Sorting and GROUP BY
Sorting and aggregation can be costly operations. Optimization tips:
- Ensure indexed columns are used in
ORDER BY
. - Use
GROUP BY
on indexed columns to reduce temporary table usage. - Avoid
ORDER BY RAND()
, which is inefficient for large datasets.
7. Limit the Number of Rows Retrieved
Using LIMIT
can significantly reduce query execution time:
SELECT name FROM customers ORDER BY created_at DESC LIMIT 10;
For pagination, use OFFSET efficiently to avoid performance degradation:
SELECT name FROM customers ORDER BY created_at DESC LIMIT 10 OFFSET 1000;
Consider an alternative approach using indexed columns instead of large OFFSET values.
8. Use Query Caching (If Applicable)
Although MySQL removed the query cache in version 8.0, application-side caching (e.g., Redis, Memcached) can improve performance for repetitive queries.
9. Batch Inserts and Updates
Performing bulk operations instead of multiple single-row statements reduces query overhead:
INSERT INTO orders (customer_id, product_id, quantity) VALUES
(1, 101, 2), (2, 102, 5), (3, 103, 1);
Similarly, update multiple rows in a single statement:
UPDATE orders SET status = 'shipped' WHERE id IN (1, 2, 3);
10. Regularly Maintain Database Health
- Analyze and optimize tables using:
ANALYZE TABLE customers;
OPTIMIZE TABLE orders;
- Monitor slow queries by enabling the slow query log:
SET GLOBAL slow_query_log = 1;
11. Timing Queries to Measure Performance Gains
To understand query performance and measure improvements, use MySQL’s SHOW PROFILE
or Benchmark()
functions.
Using SHOW PROFILE (Pre-MySQL 8.0)
SET profiling = 1;
SELECT * FROM orders WHERE customer_id = 5;
SHOW PROFILE FOR QUERY 1;
This shows execution time and CPU usage for the query.
Using Benchmark()
For quick comparisons, use:
SELECT BENCHMARK(1000000, SHA2('test', 256));
This executes a function multiple times and measures the time taken.
Using Performance Schema (MySQL 8.0+)
Enable and check query execution times:
SELECT EVENT_ID, TIMER_WAIT/1000000 AS time_ms, SQL_TEXT FROM performance_schema.events_statements_history ORDER BY EVENT_ID DESC LIMIT 5;
By timing queries before and after optimizations, you can quantify performance improvements and adjust strategies accordingly.
By applying these optimization techniques, MySQL queries can be made significantly more efficient, leading to improved database performance and scalability.