Reading time: 6 minutes.
Creating interactive and visually appealing content on your WordPress site can significantly enhance user experience. One way to achieve this is by adding a color picker that allows users to select colors and see the corresponding HEX and RGBA values. This can be useful for designers, developers, or anyone looking to offer a customizable feature on their site. In this post, I will guide you through the process of adding a simple HTML color picker with opacity control to your WordPress page.
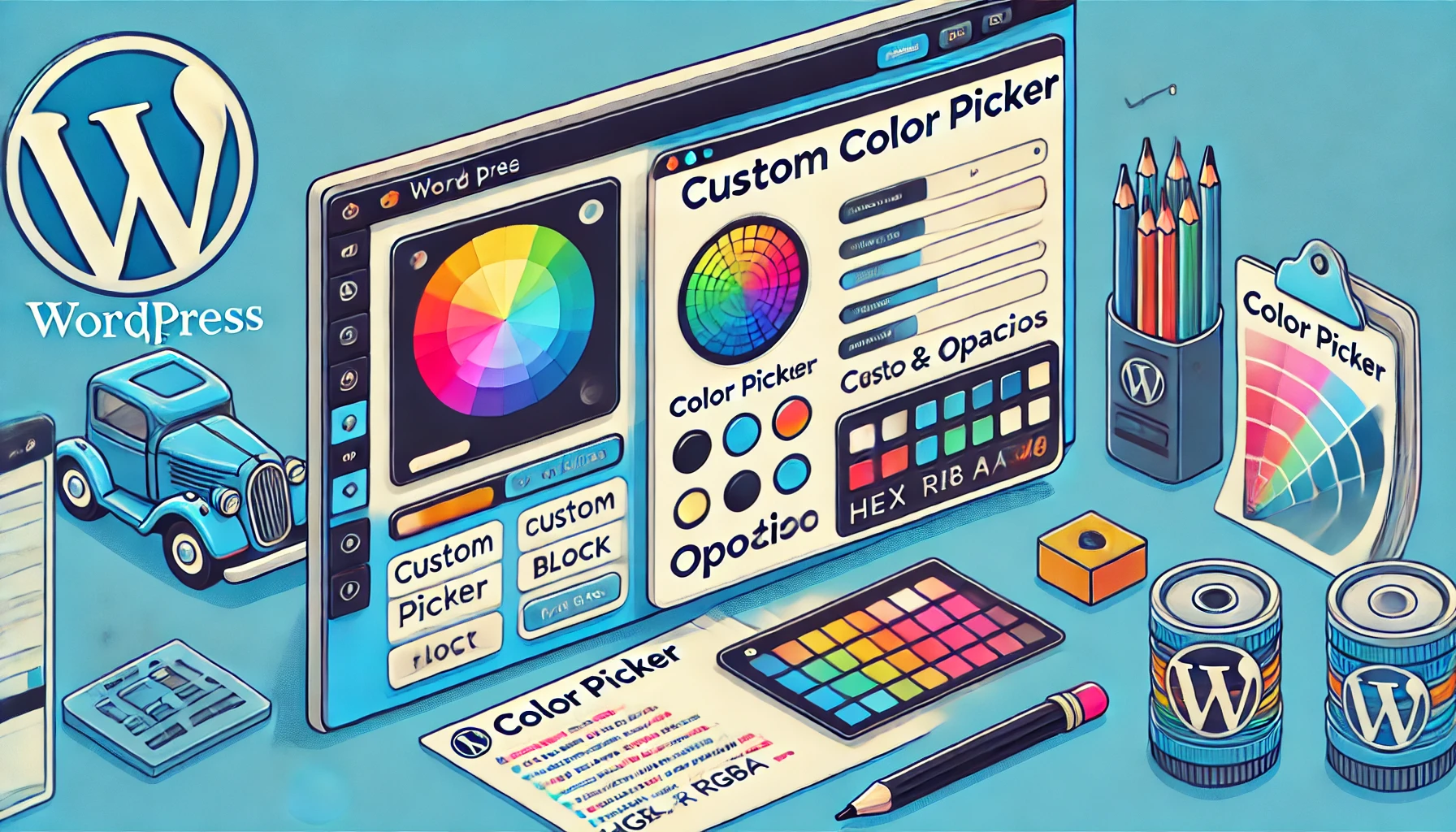
To view the simple HTML color picker, check out this page: Simple HTML Color Picker with Opacity
Step-by-Step Guide to Adding a HTML Color Picker
Step 1: Understanding the Basic Requirements
Before diving into the implementation, it’s essential to understand what we aim to achieve. We will create a color picker using HTML, CSS, and JavaScript. The color picker will include an input for selecting colors and a range slider for adjusting opacity. The selected color’s HEX and RGBA values will be displayed dynamically.
Step 2: Preparing Your WordPress Environment
Ensure you have access to the WordPress page editor. You can either use the Classic Editor or the Gutenberg (Block) Editor. This guide will focus on the Gutenberg Editor, but the steps are similar for the Classic Editor.
Step 3: Writing the HTML Code
First, let’s write the HTML code for the color picker. This code will create the basic structure of our color picker interface.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Color Picker</title>
<style>
.color-picker-container {
display: flex;
flex-direction: column;
align-items: center;
margin: 20px;
}
.color-picker {
margin: 10px;
}
.color-output {
margin-top: 20px;
}
</style>
</head>
<body>
<div class="color-picker-container">
<label for="colorPicker">Choose a color:</label>
<input type="color" id="colorPicker" class="color-picker">
<label for="opacityRange">Select opacity:</label>
<input type="range" id="opacityRange" class="color-picker" min="0" max="1" step="0.01" value="1">
<div class="color-output">
<p id="hexOutput">HEX: #000000</p>
<p id="rgbaOutput">RGBA: rgba(0, 0, 0, 1)</p>
</div>
</div>
<script>
document.addEventListener('DOMContentLoaded', function() {
const colorPicker = document.getElementById('colorPicker');
const opacityRange = document.getElementById('opacityRange');
const hexOutput = document.getElementById('hexOutput');
const rgbaOutput = document.getElementById('rgbaOutput');
function updateColor() {
const color = colorPicker.value;
const opacity = opacityRange.value;
hexOutput.textContent = `HEX: ${color}`;
const rgb = hexToRgb(color);
rgbaOutput.textContent = `RGBA: rgba(${rgb.r}, ${rgb.g}, ${rgb.b}, ${opacity})`;
}
function hexToRgb(hex) {
const bigint = parseInt(hex.slice(1), 16);
const r = (bigint >> 16) & 255;
const g = (bigint >> 8) & 255;
const b = bigint & 255;
return { r, g, b };
}
colorPicker.addEventListener('input', updateColor);
opacityRange.addEventListener('input', updateColor);
updateColor();
});
</script>
</body>
</html>
Step 4: Adding the Code to Your WordPress Page
Using the Gutenberg Editor
- Edit Your Page: Navigate to the page where you want to add the color picker. Click on “Edit” to open the Gutenberg editor.
- Add a Custom HTML Block: In the editor, click on the plus (+) icon to add a new block. Search for “Custom HTML” and add it to your page.
- Paste the HTML Code: Copy the HTML code provided above and paste it into the Custom HTML block.
- Preview Your Page: Click on “Preview” to see how the color picker looks on your page. If everything looks good, click “Update” to save your changes.
Using the Classic Editor
- Edit Your Page: Navigate to the page where you want to add the color picker. Click on “Edit” to open the Classic Editor.
- Switch to Text Editor: In the editor, switch from the “Visual” tab to the “Text” tab to access the HTML editor.
- Paste the HTML Code: Copy the HTML code provided above and paste it into the editor.
- Preview Your Page: Click on “Preview” to see how the color picker looks on your page. If everything looks good, click “Update” to save your changes.
Step 5: Enhancing the Color Picker with CSS
To make the color picker more visually appealing, you can enhance the CSS. Below is an example of additional styling you can apply to the color picker.
<style>
.color-picker-container {
display: flex;
flex-direction: column;
align-items: center;
margin: 20px;
padding: 20px;
border: 1px solid #ddd;
border-radius: 10px;
background-color: #f9f9f9;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
.color-picker {
margin: 10px;
padding: 5px;
border-radius: 5px;
}
.color-output {
margin-top: 20px;
text-align: center;
font-family: Arial, sans-serif;
color: #333;
}
#hexOutput, #rgbaOutput {
font-size: 1.2em;
margin: 5px 0;
}
</style>
You can add this CSS within the <style>
tags in the <head>
section of your HTML code or directly in your WordPress theme’s custom CSS editor.
Step 6: Adding Functionality with JavaScript
The JavaScript part of our code ensures that the color and opacity values are dynamically updated as the user interacts with the color picker and opacity range slider. Here’s a breakdown of what the JavaScript code does:
- Event Listeners: We add event listeners to the color picker and opacity range slider to detect changes.
- Update Function: The
updateColor
function updates the displayed HEX and RGBA values whenever the user selects a new color or changes the opacity. - Hex to RGB Conversion: The
hexToRgb
function converts the HEX color code to its RGB components, which are then used to construct the RGBA value.
Step 7: Testing the Color Picker
After adding the code to your WordPress page, it’s crucial to test the color picker to ensure it works as expected:
- Select Different Colors: Use the color picker to select various colors and observe the changes in the HEX and RGBA values.
- Adjust Opacity: Use the opacity range slider to adjust the opacity and verify that the RGBA values update accordingly.
- Cross-Browser Compatibility: Test the color picker in different browsers to ensure it functions correctly across all major browsers.
Step 8: Customizing and Extending the Color Picker
You can further customize and extend the functionality of the color picker based on your specific requirements. Here are a few ideas:
- Preset Colors: Add a set of preset colors that users can choose from with a single click.
- Color History: Maintain a history of selected colors for quick access.
- Color Preview: Display a preview of the selected color in a larger area for better visibility.
- Accessibility: Ensure that the color picker is accessible to users with disabilities by adding appropriate ARIA attributes and improving keyboard navigation.
Conclusion
Adding a color picker with opacity control to your WordPress page is a straightforward process that can significantly enhance the interactivity and user experience of your site. By following the steps outlined in this guide, you can create a functional and visually appealing color picker that displays both HEX and RGBA values.
This feature can be particularly useful for websites that cater to designers, developers, or any audience that requires color customization options. With some additional customization and testing, you can tailor the color picker to meet your specific needs and ensure it provides a seamless experience for your users.
Final Thoughts
As you become more comfortable with HTML, CSS, and JavaScript, you’ll find that there are endless possibilities for enhancing your WordPress site. The color picker example we’ve discussed here is just one of many interactive features you can implement to make your site more engaging and user-friendly.
Remember to always test your new features thoroughly and consider the needs of your audience. With careful planning and execution, you can create a WordPress site that not only looks great but also provides valuable functionality to your users.
Happy coding!