Reading time: 4 minutes.
JavaScript is a versatile and widely-used programming language that allows developers to perform a variety of operations on arrays. One such operation is the splice
method, which can be incredibly powerful but also somewhat confusing for beginners. This article aims to demystify the javascript splice
method by breaking down its functionality, providing clear examples, and offering tips for using it effectively.
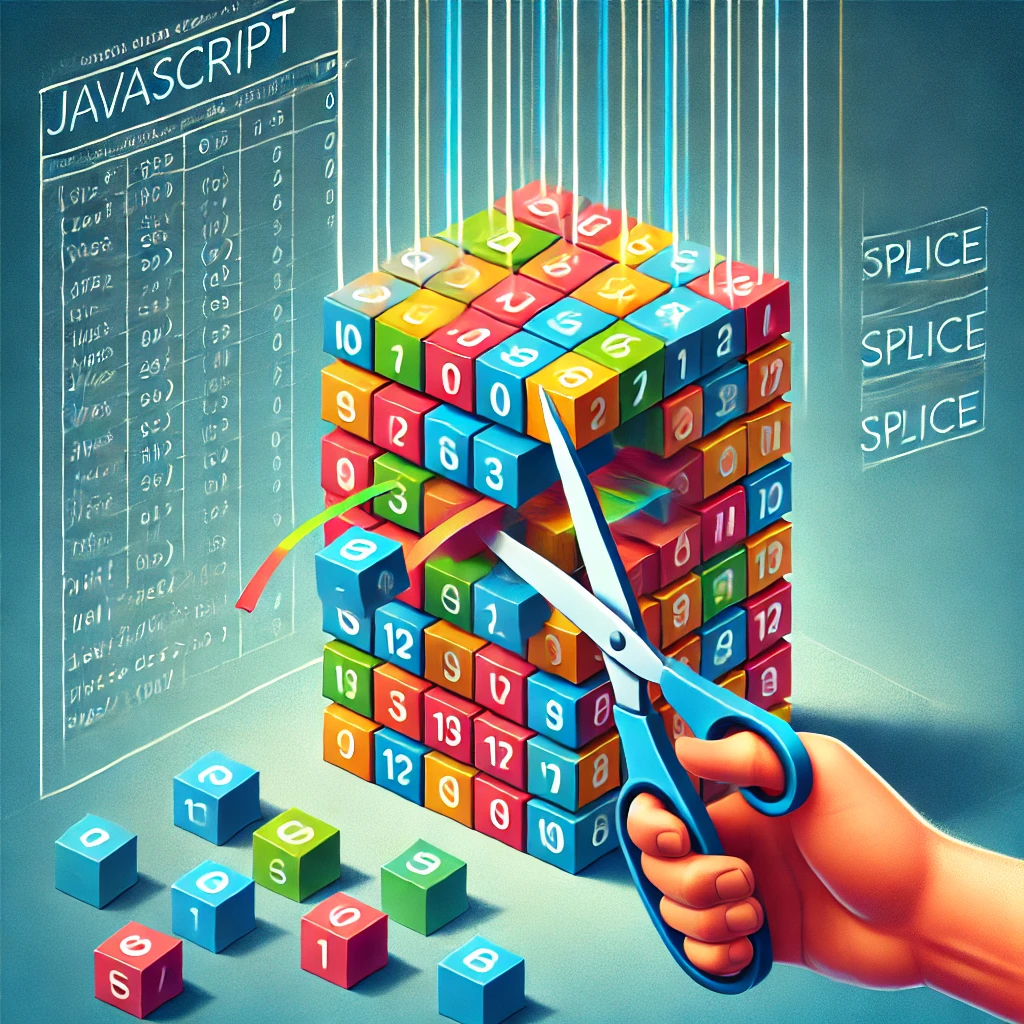
Understanding the Basics of JavaScript splice
The javascript splice
method is used to change the contents of an array by removing, replacing, or adding elements. The basic syntax of splice
is:
array.splice(start, deleteCount, item1, item2, ...);
start
: The index at which to start changing the array.deleteCount
: The number of elements to remove from the array.item1, item2, ...
: The elements to add to the array, starting from thestart
index.
Let’s break this down further with some examples.
Example 1: Removing Elements
Suppose you have an array of numbers and you want to remove a few elements from it. Here’s how you can do it with splice
:
let numbers = [1, 2, 3, 4, 5, 6];
numbers.splice(2, 3); // Removes 3 elements starting from index 2
console.log(numbers); // Output: [1, 2, 6]
In this example, splice(2, 3)
starts at index 2 and removes 3 elements (3, 4, 5
), resulting in the modified array [1, 2, 6]
.
Example 2: Adding Elements
You can also use javascript splice
to add elements to an array. Here’s an example:
let fruits = ['apple', 'banana', 'mango'];
fruits.splice(1, 0, 'orange', 'pineapple'); // Adds 'orange' and 'pineapple' at index 1
console.log(fruits); // Output: ['apple', 'orange', 'pineapple', 'banana', 'mango']
In this case, splice(1, 0, 'orange', 'pineapple')
starts at index 1, removes 0 elements, and adds 'orange'
and 'pineapple'
, resulting in the modified array ['apple', 'orange', 'pineapple', 'banana', 'mango']
.
Example 3: Replacing Elements
The splice
method can also be used to replace elements in an array. For example:
let animals = ['cat', 'dog', 'mouse'];
animals.splice(1, 1, 'rabbit', 'hamster'); // Replaces 'dog' with 'rabbit' and 'hamster'
console.log(animals); // Output: ['cat', 'rabbit', 'hamster', 'mouse']
Here, splice(1, 1, 'rabbit', 'hamster')
starts at index 1, removes 1 element ('dog'
), and adds 'rabbit'
and 'hamster'
, resulting in the modified array ['cat', 'rabbit', 'hamster', 'mouse']
.
Practical Use Cases for JavaScript splice
Removing Specific Items from an Array
One common use case for splice
is removing specific items from an array. For instance, if you have an array of user objects and you want to remove a user with a specific ID, you can do it like this:
let users = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 3, name: 'Charlie' }
];
let userIdToRemove = 2;
let index = users.findIndex(user => user.id === userIdToRemove);
if (index !== -1) {
users.splice(index, 1);
}
console.log(users); // Output: [{ id: 1, name: 'Alice' }, { id: 3, name: 'Charlie' }]
In this example, findIndex
is used to find the index of the user with ID 2. Then, splice
is used to remove that user from the array.
Inserting Elements at a Specific Position
Another practical use of splice
is inserting elements at a specific position in an array. For example, if you have an ordered list and you want to insert a new item at a specific position:
let orderedList = ['item1', 'item2', 'item4'];
let newItem = 'item3';
let position = 2;
orderedList.splice(position, 0, newItem);
console.log(orderedList); // Output: ['item1', 'item2', 'item3', 'item4']
In this case, splice(2, 0, 'item3')
inserts 'item3'
at index 2 without removing any elements.
Replacing Elements Conditionally
You can also use javascript splice
to replace elements conditionally. For example, if you have a list of products and you want to update the price of a specific product:
let products = [
{ id: 1, name: 'Laptop', price: 1000 },
{ id: 2, name: 'Tablet', price: 500 },
{ id: 3, name: 'Smartphone', price: 700 }
];
let productIdToUpdate = 2;
let newPrice = 550;
let index = products.findIndex(product => product.id === productIdToUpdate);
if (index !== -1) {
products.splice(index, 1, { ...products[index], price: newPrice });
}
console.log(products); // Output: [{ id: 1, name: 'Laptop', price: 1000 }, { id: 2, name: 'Tablet', price: 550 }, { id: 3, name: 'Smartphone', price: 700 }]
In this example, findIndex
is used to find the index of the product with ID 2. Then, splice
is used to replace the product object with a new object that has the updated price.
Tips for Using JavaScript splice Effectively
- Understand the Indexing: Remember that array indexing starts at 0. This is crucial for correctly specifying the
start
parameter. - Check Index Validity: Always ensure the index you are using for
splice
is within the bounds of the array to avoid unexpected results. - Use
splice
for In-place Modifications: Thesplice
method modifies the original array. If you need to keep the original array unchanged, consider using other methods likeslice
to create a copy before splicing. - Be Mindful of Performance: Splicing large arrays can be computationally expensive. If performance is a concern, explore other options or optimize your code to minimize splicing operations.
- Combine with Other Methods:
splice
can be powerful when combined with other array methods likefindIndex
,filter
, andmap
for more complex operations.
Conclusion
The splice
method in JavaScript is a versatile tool for manipulating arrays. By understanding its basic syntax and various use cases, you can leverage its power to perform a wide range of operations on arrays. Whether you need to remove, add, or replace elements, splice
provides a straightforward way to achieve your goal. With the tips provided, you can use javascript splice
effectively and efficiently in your JavaScript projects.
Remember, practice makes perfect. Experiment with different splice
operations and see how they affect your arrays. As you become more comfortable with javascript splice
, you’ll find it to be an indispensable part of your JavaScript toolkit.