Reading time: 6 minutes.
Introduction
In the modern web development landscape, creating responsive and adaptive web designs has become paramount. With the proliferation of devices of various screen sizes and resolutions, from smartphones and tablets to desktops and large monitors, ensuring that a website looks and functions well on all these devices is crucial. CSS media queries are one of the most powerful tools available to web developers for achieving responsive design. This article will delve deep into CSS media queries, exploring their syntax, usage, and best practices, while providing practical examples to illustrate their effectiveness.
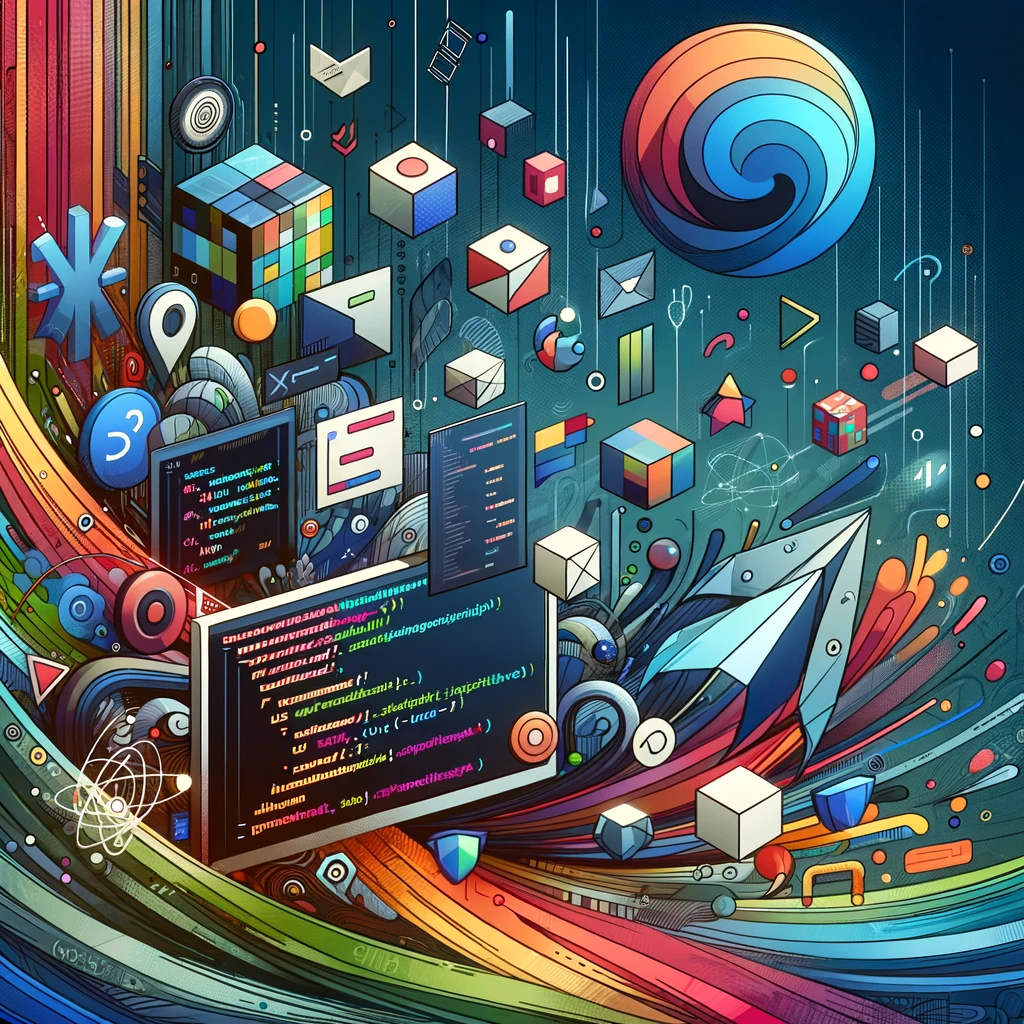
What are CSS Media Queries?
CSS media queries are a feature of CSS3 that allow developers to apply different styles to a website or web application based on the characteristics of the user’s device, such as screen width, height, resolution, orientation, and more. Media queries enable the creation of a responsive web design, where the layout and content of a site adapt to the device’s specifications, providing an optimal user experience across a wide range of devices.
Syntax of CSS Media Queries
The basic syntax of a media query is as follows:
@media (media-type) and (media-feature) {
/* CSS rules */
}
- @media: This is the keyword that starts a media query.
- media-type: This specifies the type of media (e.g., screen, print, all).
- media-feature: This defines the specific feature or features to query (e.g., min-width, max-width, orientation).
Here’s a simple example:
@media screen and (max-width: 600px) {
body {
background-color: lightblue;
}
}
In this example, the background color of the body will change to light blue when the screen width is 600 pixels or less.
Media Types
Media types allow developers to specify the kind of device for which the styles are intended. The most commonly used media types are:
- all: Suitable for all devices.
- print: Intended for printed materials and documents displayed in a print preview.
- screen: Used for computer screens, tablets, smartphones, and other screen-based devices.
- speech: Intended for screen readers.
Example:
@media print {
body {
font-size: 12pt;
}
}
This media query applies a font size of 12 points when the content is printed.
Media Features
Media features are the conditions that a device must meet for the styles to be applied. Some common media features include:
- width: The width of the viewport.
- height: The height of the viewport.
- device-width: The width of the device’s screen.
- device-height: The height of the device’s screen.
- orientation: The orientation of the device (portrait or landscape).
- resolution: The resolution of the device’s screen.
- aspect-ratio: The ratio of the width to the height of the viewport.
Min and Max Prefixes
The min-
and max-
prefixes are often used with media features to specify minimum and maximum values, respectively. For example:
- min-width: Specifies a minimum width.
- max-width: Specifies a maximum width.
- min-height: Specifies a minimum height.
- max-height: Specifies a maximum height.
Example:
@media screen and (min-width: 768px) {
.container {
width: 750px;
}
}
This media query applies the styles inside the block when the viewport width is at least 768 pixels.
Practical Examples of CSS Media Queries
Responsive Typography
One of the simplest and most effective uses of media queries is adjusting font sizes based on the viewport width. This ensures readability across devices.
body {
font-size: 16px;
}
@media screen and (max-width: 600px) {
body {
font-size: 14px;
}
}
@media screen and (min-width: 1200px) {
body {
font-size: 18px;
}
}
In this example, the base font size is set to 16px. If the viewport width is 600px or less, the font size is reduced to 14px. If the viewport width is 1200px or more, the font size is increased to 18px.
Layout Adjustments
Adjusting the layout based on the screen size is a common use of media queries. For instance, changing a multi-column layout to a single-column layout on smaller screens can enhance usability.
.container {
display: flex;
flex-direction: row;
}
@media screen and (max-width: 768px) {
.container {
flex-direction: column;
}
}
Here, the .container
class initially displays its children in a row. When the viewport width is 768px or less, the direction changes to a column.
Hiding and Showing Content
Sometimes, it is necessary to hide certain elements on smaller screens to improve the user experience.
.navbar {
display: flex;
}
.mobile-menu {
display: none;
}
@media screen and (max-width: 768px) {
.navbar {
display: none;
}
.mobile-menu {
display: block;
}
}
In this example, the .navbar
is displayed by default, and the .mobile-menu
is hidden. When the viewport width is 768px or less, the .navbar
is hidden, and the .mobile-menu
is displayed.
Advanced CSS Media Query Techniques
Combining Multiple Conditions
Media queries can combine multiple conditions using logical operators such as and
, not
, and only
.
Using and
@media screen and (min-width: 600px) and (max-width: 1200px) {
.container {
background-color: lightgreen;
}
}
This media query applies the styles inside the block when the viewport width is between 600px and 1200px.
Using not
@media not all and (min-width: 600px) {
.container {
background-color: lightcoral;
}
}
This media query applies the styles inside the block when the viewport width is less than 600px.
Using only
The only
operator prevents older browsers that do not support media queries from applying the styles.
@media only screen and (min-width: 600px) {
.container {
background-color: lightgoldenrodyellow;
}
}
Media Queries for High-Resolution Devices
To ensure that your site looks good on high-resolution devices, such as those with Retina displays, you can use the resolution
media feature.
@media screen and (min-resolution: 2dppx) {
.high-res-image {
background-image: url('high-res-image.png');
}
}
In this example, the .high-res-image
class applies a different background image for devices with a resolution of 2 device pixels per CSS pixel or higher.
Best Practices for Using CSS Media Queries
Mobile-First Approach
A mobile-first approach involves designing for the smallest screens first and then progressively enhancing the design for larger screens. This approach ensures that the base design is optimized for mobile devices.
/* Base styles for mobile devices */
.container {
width: 100%;
}
/* Styles for larger screens */
@media screen and (min-width: 768px) {
.container {
width: 750px;
}
}
@media screen and (min-width: 1200px) {
.container {
width: 1170px;
}
}
Keep Media Queries Organized
Organizing your media queries can make your CSS more maintainable. You can group media queries by breakpoints or place them alongside the related CSS rules.
Grouping by Breakpoints
/* Base styles */
.container {
width: 100%;
}
/* Breakpoint for tablets */
@media screen and (min-width: 768px) {
.container {
width: 750px;
}
}
/* Breakpoint for desktops */
@media screen and (min-width: 1200px) {
.container {
width: 1170px;
}
}
Placing Media Queries with Related Rules
.container {
width: 100%;
}
@media screen and (min-width: 768px) {
.container {
width: 750px;
}
}
@media screen and (min-width: 1200px) {
.container {
width: 1170px;
}
}
Test Across Multiple Devices
Always test your designs on multiple devices and screen sizes to ensure that your media queries are working as intended. Emulators and responsive design testing tools can be very helpful in this regard.
Conclusion
CSS media queries are an essential tool for creating responsive web designs. They allow developers to apply different styles based on the characteristics of the user’s device, ensuring an optimal user experience across a wide range of devices. By understanding the syntax, usage, and best practices of media queries, you can create flexible and adaptive designs that look great on any screen size.
As you continue to build your CSS skills, remember to experiment with different media features and combinations, and always keep the user experience in mind. With practice, you’ll be able to leverage the full power of CSS media queries to create stunning, responsive web designs.