Reading time: 2 minutes.
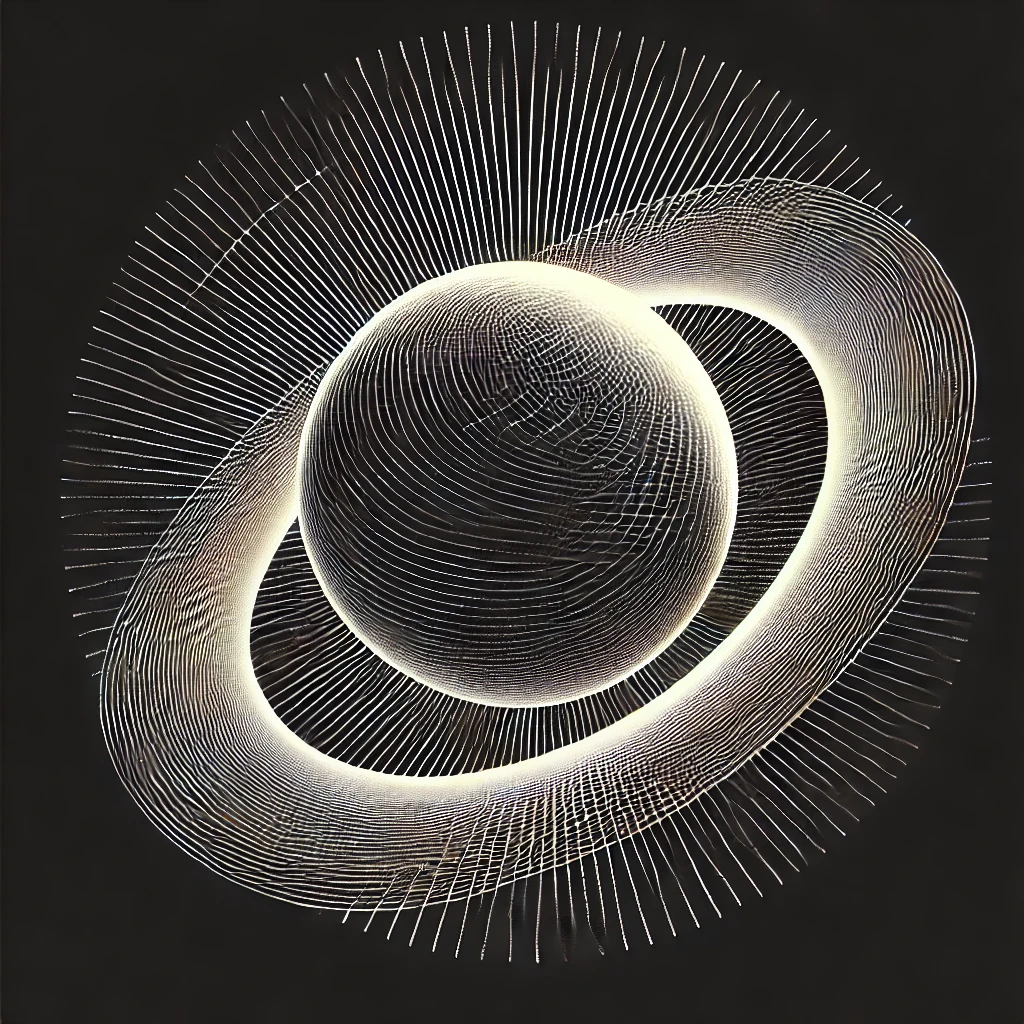
CSS makes it easy to create animations, including a spinning image effect. Whether you want a simple 2D rotation or a more dynamic 3D perspective, CSS provides the tools to achieve this. Animations in CSS are achieved using the @keyframes
rule, which allows elements to transition between different states over a specified duration. This technique is widely used in web design to create engaging visual effects, improve user interaction, and make interfaces feel more dynamic.
In this guide, we will cover two ways to create a spinning image. First, we’ll explore a simple 2D rotation using rotate()
, which is ideal for basic animations. Then, we will introduce a 3D spinning effect using rotateY()
along with perspective to create a more immersive visual. These methods can be used in various scenarios, from loading animations to interactive UI elements.
Basic Spinning Image (2D Rotation)
To create a simple spinning image, we use the CSS @keyframes
rule to define the rotation and apply it using the animation
property.
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Spinning Image</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="container">
<img src="image.jpg" alt="Spinning Image" class="spin-image">
</div>
</body>
</html>
CSS:
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #f0f0f0;
}
.container {
width: 200px;
height: 200px;
}
.spin-image {
width: 100%;
height: auto;
animation: spin 3s linear infinite;
}
@keyframes spin {
from {
transform: rotate(0deg);
}
to {
transform: rotate(360deg);
}
}
This will continuously rotate the image around its center.
Adding Perspective (3D Rotation)
For a more dynamic effect, we can add a 3D perspective using the transform
property with rotateY
.
Updated CSS for 3D Spinning Effect:
.spin-image {
width: 100%;
height: auto;
transform-style: preserve-3d;
animation: spin3d 3s linear infinite;
}
@keyframes spin3d {
from {
transform: rotateY(0deg);
}
to {
transform: rotateY(360deg);
}
}
This will create a 3D effect where the image appears to spin around a vertical axis.
Adding Perspective to the Container
To enhance the 3D effect, we can wrap the image inside a container with a defined perspective
.
Updated CSS:
.container {
perspective: 500px;
width: 200px;
height: 200px;
}
.spin-image {
transform-origin: center;
animation: spin3d 3s linear infinite;
}
With perspective, the spinning effect appears more realistic, as if the image is rotating in 3D space.
Conclusion
If you want to see this in action, check out this codepen.
Using CSS animations, you can create simple or advanced spinning image effects. The 2D rotation works well for basic needs, while the 3D version with perspective adds depth and realism. Try tweaking the perspective and animation speed to suit your needs!