Reading time: 5 minutes.
In today’s digital age, where screen time is increasing, the demand for dark mode in applications has skyrocketed. Dark mode not only reduces eye strain in low-light environments but also conserves battery life on devices with OLED screens. Implementing dark mode can enhance user experience significantly. This article will guide you through adding dark mode to your application using only CSS, making it accessible and comfortable for night-time users.
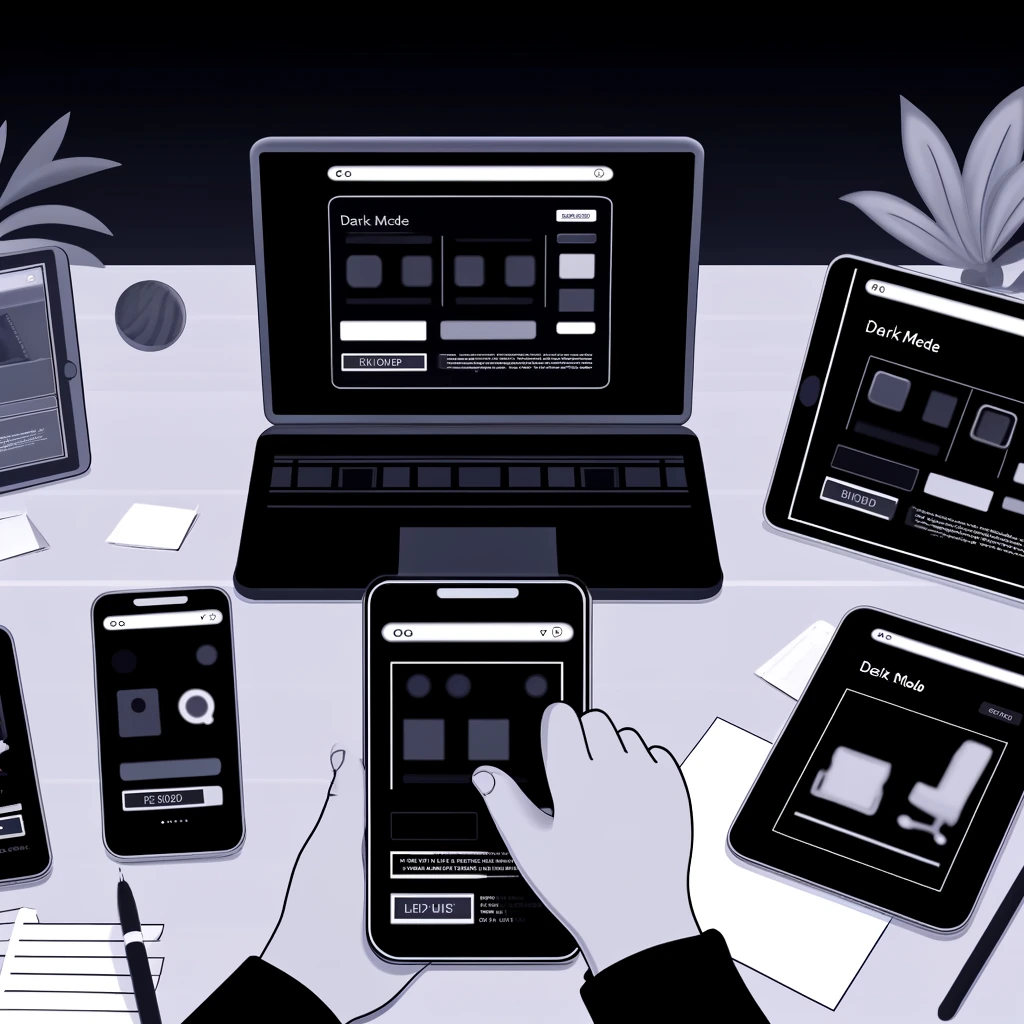
Understanding Dark Mode
Dark mode is a feature that changes the UI of an application to a darker theme. This is not just about aesthetics but also about usability and accessibility. Many users prefer dark mode for its visual comfort and minimalistic style.
Step 1: Planning Your Color Palette
Before you start coding, it’s essential to plan your color palette. You need to decide on the colors for both light and dark themes. Here’s a simple guide on choosing colors:
- Background Colors: Choose darker shades for the background in dark mode. Common choices are shades of black or very dark grays.
- Text Colors: Text should be lighter in dark mode. White or light gray can work well.
- Accent Colors: Accent colors should be less saturated. Bright colors often used in light mode can be overwhelming in dark mode.
Step 2: Using CSS Custom Properties
CSS Custom Properties (also known as CSS Variables) are perfect for implementing theming. They allow you to define a value once and use it in multiple places. Here’s how to define your colors:
:root {
/* Light theme colors */
--background-color: #ffffff;
--text-color: #333333;
--accent-color: #0066ff;
/* Dark theme colors */
--background-color-dark: #121212;
--text-color-dark: #cccccc;
--accent-color-dark: #3399ff;
}
Step 3: Toggling Themes with a CSS Class
Now, let’s use these variables to apply the themes. We will switch between light and dark themes using a class on the <body>
tag.
body {
background-color: var(--background-color);
color: var(--text-color);
}
body.dark {
--background-color: var(--background-color-dark);
--text-color: var(--text-color-dark);
--accent-color: var(--accent-color-dark);
}
This approach modifies the CSS variables when the .dark
class is applied, effectively changing the theme.
Step 4: Adding a Theme Toggle Button
To let users switch between themes, we need a toggle. Here’s a simple button implementation:
<button onclick="toggleTheme()">Toggle Dark Mode</button>
And the corresponding JavaScript:
function toggleTheme() {
document.body.classList.toggle('dark');
}
This script toggles the dark
class on the <body>
, switching the theme.
Step 5: Using Media Queries for Automatic Detection
Some users have set their operating systems to use dark mode. We can automatically match this preference using CSS media queries:
@media (prefers-color-scheme: dark) {
body {
--background-color: var(--background-color-dark);
--text-color: var(--text-color-dark);
--accent-color: var(--accent-color-dark);
}
}
This method automatically applies dark mode if the user has set their system preference to dark.
Step 6: Ensuring Accessibility
When implementing dark mode, ensure your application remains accessible. Contrast ratios should meet WCAG (Web Content Accessibility Guidelines) standards. Tools like the WebAIM Contrast Checker can help verify your color choices.
Step 7: Testing Across Devices and Browsers
Testing is crucial. Check your dark mode on various devices and browsers to ensure it looks consistent and functions well. Pay particular attention to how images, icons, and other elements appear in dark mode.
An Amazing Hack to Add Dark Mode
For developers looking for a quick and somewhat unconventional way to implement dark mode, CSS provides a fascinating property: filter
. Using the filter
property with the invert(1)
value can instantly transform a light-themed website into dark mode. This method is not commonly recommended for production environments due to its broad and somewhat unpredictable effects, but it can serve as a great starting point or quick demonstration.
How Does filter: invert(1)
Work?
The invert(1)
filter inverts all the colors of the elements it is applied to. When applied at a full value of 1
, it completely inverts the colors, which means black becomes white, blue becomes orange, and so forth. This creates an effect that, at first glance, closely resembles a dark mode.
Implementing filter: invert(1)
To apply this method, you can add a class that toggles the invert filter on the body element:
body.dark-mode {
filter: invert(1) hue-rotate(180deg);
}
Here, hue-rotate(180deg)
is also used along with invert(1)
. The hue rotation adjusts the colors back towards their original hue after inversion, which helps maintain some of the original color relationships and aesthetics.
JavaScript Toggle
Similar to the previous method, you can use a toggle button to add or remove the dark-mode
class from the body:
<button onclick="toggleDarkMode()">Toggle Dark Mode</button>
And the JavaScript function:
function toggleDarkMode() {
document.body.classList.toggle('dark-mode');
}
Considerations and Limitations
While using filter: invert(1)
is a quick and easy hack, it comes with significant drawbacks:
- Images and Media: This method inverts everything, including images, videos, and icons, which might not be desirable. Images may appear with incorrect colors and can be disorienting.
- Performance: Applying a filter to the entire body can lead to performance issues, particularly on less powerful devices or with complex web applications.
- Fine-Tuning: It’s a blunt tool that doesn’t allow for fine-tuned theming. For example, specific colors that should not invert (like logos or specific UI elements) can’t be controlled without additional CSS overrides, which can complicate the stylesheet.
- Accessibility: Inverting colors can also inadvertently create accessibility issues, especially if the resulting contrast ratios are not compliant with WCAG guidelines.
When to Use This Hack
This approach can be beneficial for:
- Quick prototypes: When you need to demonstrate a dark mode without implementing a full theme.
- Personal use: If you’re using a browser extension or a personal script to invert colors on websites you visit.
Conclusion
The filter: invert(1)
hack for dark mode is an interesting CSS feature that showcases the powerful capabilities of CSS filters. However, for a more professional and polished dark mode implementation, it’s advisable to use the earlier described method of CSS custom properties with thoughtful color scheming. This ensures a more consistent, performant, and accessible user experience.
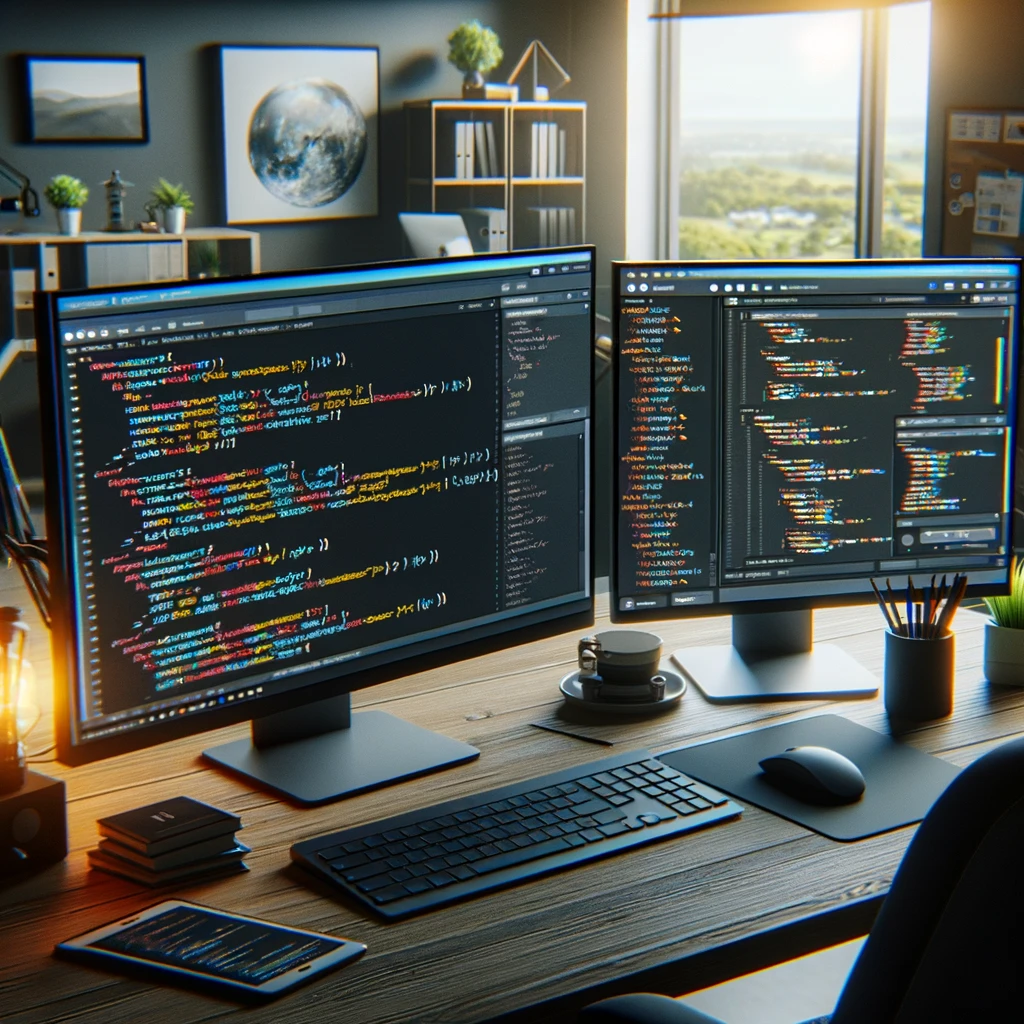
Using Javascript to Detect Dark Mode Preference
If you require a means to test for Dark Mode in JS, you can use:
if (window.matchMedia && window.matchMedia('(prefers-color-scheme: dark)').matches) {
// dark mode handler
}
What if your users change their preference? To listen for changes, use this event listener:
window.matchMedia('(prefers-color-scheme: dark)').addEventListener('change', event => {
const newColorScheme = event.matches ? "dark" : "light";
});
Wrapping Up
Implementing dark mode using CSS is a straightforward and effective way to enhance your application’s user experience. By following these steps, you can offer a visually appealing and eye-friendly alternative to traditional light-themed interfaces. Remember, the key to a successful implementation is careful planning, consistent testing, and thoughtful consideration of accessibility standards.
Implementing dark mode isn’t just about following trends; it’s about improving usability and accessibility for all users, regardless of their environment or preferences. By integrating dark mode into your application, you’re taking a significant step towards a more inclusive digital world.