Reading time: 4 minutes.
In the evolving landscape of JavaScript, new features and operators are introduced to make coding more intuitive and efficient. One such feature is the double question mark (??
), also known as the nullish coalescing operator. This operator has gained popularity for its simplicity and effectiveness in handling nullish values. In this post, we’ll dive into the amazing use cases of the JavaScript double question mark, exploring how it can simplify your code and improve readability.
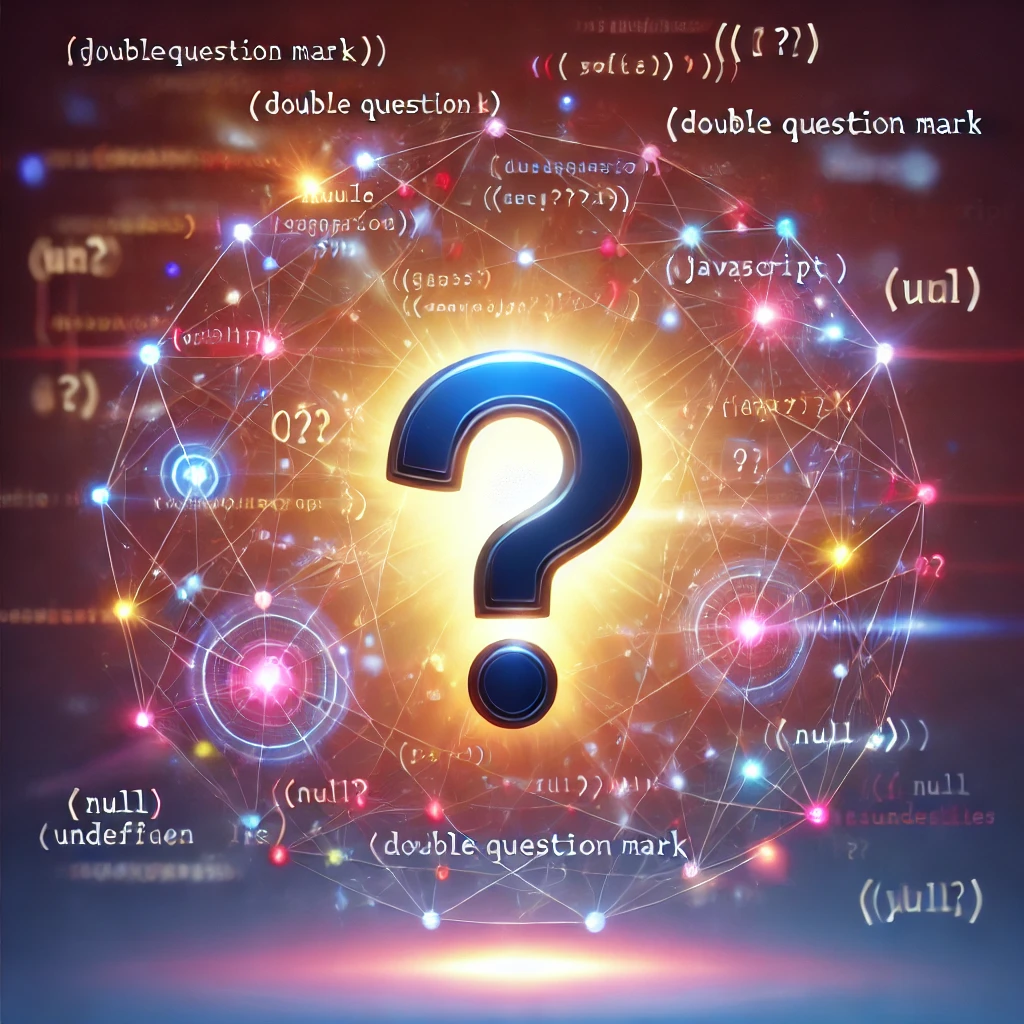
Understanding the JavaScript Double Question Mark and the Nullish Coalescing Operator
Before delving into the use cases, let’s first understand what the nullish coalescing operator is and how it works. The double question mark (??
) is a logical operator that returns the right-hand operand when the left-hand operand is null
or undefined
. Otherwise, it returns the left-hand operand.
Here’s the basic syntax:
let result = a ?? b;
In this expression, result
will be a
if a
is not null
or undefined
; otherwise, it will be b
.
Comparison with Logical OR (||
)
Many developers might wonder how ??
differs from the logical OR operator (||
). While both operators can be used for default values, they behave differently:
||
returns the right-hand operand if the left-hand operand is falsy (i.e.,false
,0
,NaN
,""
,null
, orundefined
).??
returns the right-hand operand only if the left-hand operand isnull
orundefined
.
Here’s a quick comparison:
let a = 0;
let b = a || 10; // b will be 10 because 0 is falsy
let c = a ?? 10; // c will be 0 because a is not null or undefined
Amazing Use Cases of the Javascript Double Question Mark
Now that we understand the basics, let’s explore some practical use cases where the nullish coalescing operator shines.
1. JavaScript Double Question Mark and Default Values
One of the most common use cases of ??
is to provide default values for variables that might be null
or undefined
.
function greet(name) {
let userName = name ?? "Guest";
console.log(`Hello, ${userName}!`);
}
greet("Alice"); // Output: Hello, Alice!
greet(null); // Output: Hello, Guest!
greet(); // Output: Hello, Guest!
In this example, if name
is not provided or is null
, userName
defaults to “Guest”.
2. JavaScript Double Question Mark and Simplifying Optional Chaining
When combined with optional chaining (?.
), ??
can handle deeply nested objects with more grace.
let user = {
profile: {
email: "user@example.com"
}
};
let email = user.profile?.email ?? "No email provided";
console.log(email); // Output: user@example.com
let phone = user.profile?.phone ?? "No phone number provided";
console.log(phone); // Output: No phone number provided
This approach avoids errors when accessing properties of objects that might not exist, providing a default value instead.
3. Use JavaScript Double Question Mark to Handle Function Parameters
The nullish coalescing operator is particularly useful in function parameters, ensuring that parameters have meaningful default values.
function createUser(name, age) {
let userName = name ?? "Anonymous";
let userAge = age ?? 18;
return {
name: userName,
age: userAge
};
}
console.log(createUser("Bob", 25)); // Output: { name: 'Bob', age: 25 }
console.log(createUser(null, null)); // Output: { name: 'Anonymous', age: 18 }
In this example, name
and age
default to “Anonymous” and 18 if not provided.
4. Working with API Responses
When working with data from APIs, properties might be missing or null
. Using ??
, you can set default values to ensure your application behaves predictably.
fetch('https://api.example.com/user/1')
.then(response => response.json())
.then(data => {
let userName = data.name ?? "Unknown User";
let userEmail = data.email ?? "No email provided";
console.log(`Name: ${userName}, Email: ${userEmail}`);
});
This ensures that even if the API response doesn’t include name
or email
, the application will not break and will show default values instead.
5. Configuring Application Settings with the JavaScript Double Question Mark
For applications with configurable settings, ??
can provide default values when settings are not specified.
const config = {
theme: "dark",
language: null
};
let theme = config.theme ?? "light";
let language = config.language ?? "en";
console.log(`Theme: ${theme}, Language: ${language}`); // Output: Theme: dark, Language: en
This makes your application more robust and user-friendly by ensuring it has sensible defaults.
6. Ensuring Safe Math Operations
When performing math operations, it’s essential to ensure that the values are not null
or undefined
. The ??
operator can help in such scenarios.
function add(a, b) {
return (a ?? 0) + (b ?? 0);
}
console.log(add(5, null)); // Output: 5
console.log(add(undefined, 3)); // Output: 3
console.log(add(null, undefined)); // Output: 0
By defaulting to 0, you avoid unexpected results from nullish values.
7. Improving Code Readability with the JavaScript Double Question Mark
Using ??
can make your code more readable and expressive, clearly indicating that you are dealing with null
or undefined
values.
let userInput = getInput() ?? "Default Value";
This line of code immediately communicates that userInput
should default to “Default Value” if getInput()
returns null
or undefined
.
8. Simplifying State Management
In state management, particularly with frameworks like React, ??
can be used to provide default state values, making your components more robust.
const [count, setCount] = useState(null);
const displayCount = count ?? 0;
return <div>Count: {displayCount}</div>;
Here, the component displays 0
if count
is null
, ensuring a consistent UI.
9. Handling Form Inputs with JavaScript Double Question Mark
When managing form inputs, ??
can be used to handle missing or undefined values gracefully.
let formData = {
name: "",
age: undefined
};
let name = formData.name ?? "Unknown";
let age = formData.age ?? "N/A";
console.log(`Name: ${name}, Age: ${age}`); // Output: Name: Unknown, Age: N/A
This approach ensures that your form handling logic is robust and user-friendly.
How Will You Use the JavaScript Double Question Mark?
The nullish coalescing operator (??
) is a powerful and concise tool for handling null
and undefined
values in JavaScript. Its primary use case is to provide default values, making your code more robust, readable, and efficient. Whether you’re dealing with function parameters, API responses, state management, or form inputs, ??
offers a straightforward solution to ensure your code behaves predictably in the face of nullish values.
As you incorporate ??
into your JavaScript toolkit, you’ll likely find more scenarios where it simplifies your code and improves overall code quality. Embrace the power of the double question mark and enjoy the benefits of cleaner and more maintainable JavaScript code.