Reading time: 5 minutes.
Creating a pension calculator for your WordPress page can be a valuable tool for your visitors, allowing them to plan their retirement savings efficiently. By integrating a simple and interactive calculator, you can help users estimate their future savings based on their current age, retirement age, current savings, monthly contributions, and expected annual return. This guide will walk you through creating a simple pension calculator using HTML, CSS, and JavaScript. The provided code can be easily embedded into your WordPress page, making it accessible to your audience.
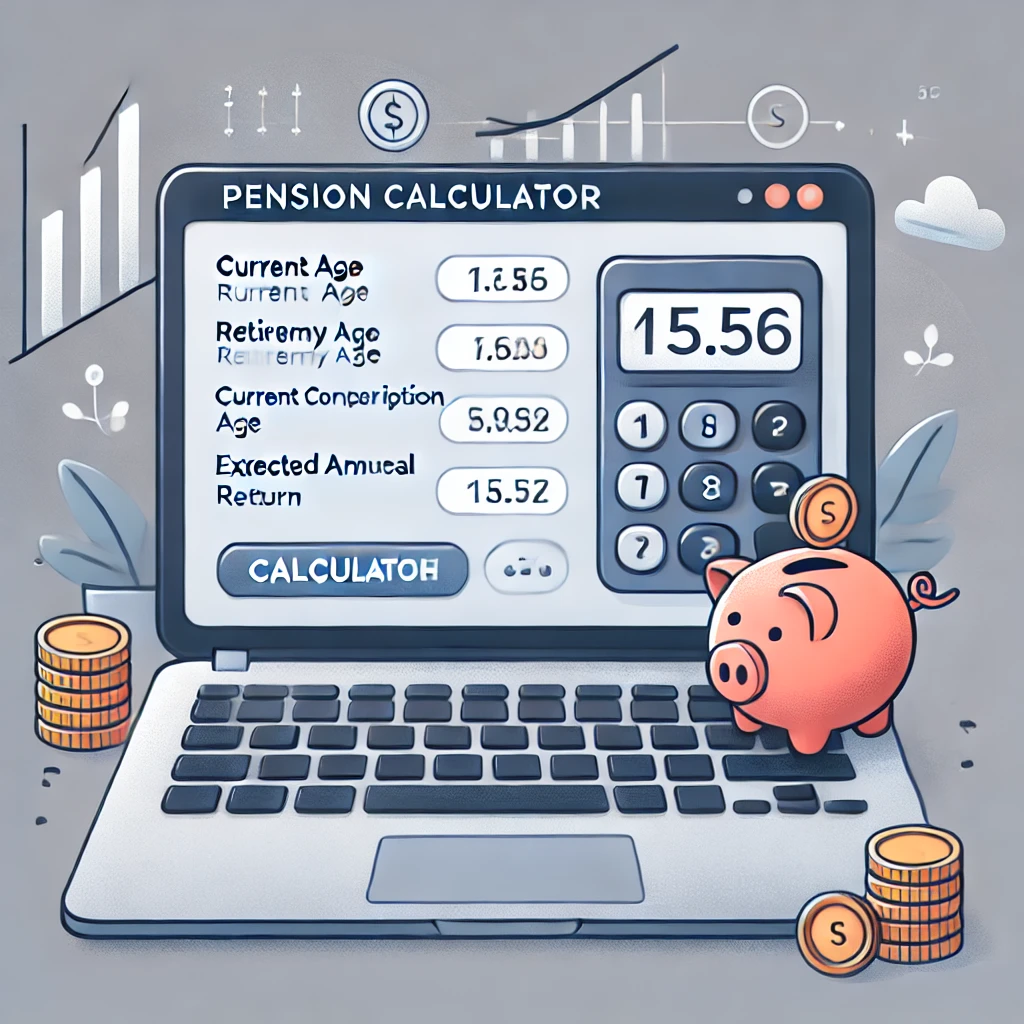
To check out the Simple Online Pension Calculator I made, check this out: Simple Online Pension Calculator
Why Create a Pension Calculator?
Retirement planning is crucial for financial stability in the later stages of life. Many individuals struggle to estimate how much they need to save to maintain their lifestyle after retirement. A pension calculator provides a clear and concise way to project future savings, helping users make informed decisions about their financial planning.
Steps to Create the Pension Calculator
1. Prepare Your WordPress Environment
Before adding the pension calculator, ensure your WordPress environment is set up correctly. You need administrative access to create and edit pages or posts where you will embed the calculator.
2. Create a New Page or Post
Navigate to your WordPress dashboard, and create a new page or post where you want the pension calculator to appear. You can name it something descriptive, like “Pension Calculator.”
3. Add the HTML and JavaScript Code
In your newly created page or post, switch to the HTML editor. If you are using the Block Editor (Gutenberg), add a “Custom HTML” block. If you are using the Classic Editor, switch to the “Text” tab. Copy and paste the following code into the editor:
<!DOCTYPE html>
<html>
<head>
<style>
.calculator-container {
max-width: 400px;
margin: auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 10px;
background-color: #f9f9f9;
}
.calculator-container h2 {
text-align: center;
}
.calculator-container label {
display: block;
margin: 15px 0 5px;
}
.calculator-container input {
width: 100%;
padding: 10px;
margin-bottom: 10px;
border: 1px solid #ccc;
border-radius: 5px;
}
.calculator-container button {
width: 100%;
padding: 10px;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
.calculator-container button:hover {
background-color: #45a049;
}
.calculator-container .result {
margin-top: 20px;
padding: 10px;
border: 1px solid #ccc;
border-radius: 5px;
background-color: #e9e9e9;
text-align: center;
}
</style>
</head>
<body>
<div class="calculator-container">
<h2>Pension Calculator</h2>
<label for="age">Current Age:</label>
<input type="number" id="age" min="18" max="100" value="30">
<label for="retirementAge">Retirement Age:</label>
<input type="number" id="retirementAge" min="50" max="100" value="65">
<label for="currentSavings">Current Savings ($):</label>
<input type="number" id="currentSavings" min="0" value="10000">
<label for="monthlyContribution">Monthly Contribution ($):</label>
<input type="number" id="monthlyContribution" min="0" value="200">
<label for="annualReturn">Expected Annual Return (%):</label>
<input type="number" id="annualReturn" min="0" max="100" value="5">
<button onclick="calculatePension()">Calculate</button>
<div class="result" id="result"></div>
</div>
<script>
function calculatePension() {
// Get user input
const age = parseFloat(document.getElementById('age').value);
const retirementAge = parseFloat(document.getElementById('retirementAge').value);
const currentSavings = parseFloat(document.getElementById('currentSavings').value);
const monthlyContribution = parseFloat(document.getElementById('monthlyContribution').value);
const annualReturn = parseFloat(document.getElementById('annualReturn').value) / 100;
// Calculate years until retirement
const yearsUntilRetirement = retirementAge - age;
// Initialize total savings
let totalSavings = currentSavings;
// Calculate total savings at retirement
for (let i = 0; i < yearsUntilRetirement; i++) {
totalSavings += monthlyContribution * 12;
totalSavings *= (1 + annualReturn);
}
// Display the result
document.getElementById('result').innerText = `Total Savings at Retirement: $${totalSavings.toFixed(2)}`;
}
</script>
</body>
</html>
4. Publish the Page or Post
Once you have pasted the code, save your changes and publish the page or post. Your pension calculator is now live and ready for use.
Understanding the Code
The pension calculator consists of three main components: HTML for structure, CSS for styling, and JavaScript for functionality.
HTML Structure
The HTML structure defines the layout of the calculator. It includes input fields for the user to enter their current age, retirement age, current savings, monthly contributions, and expected annual return. A button triggers the calculation, and a div
element displays the result.
CSS Styling
The CSS styling enhances the appearance of the calculator, making it user-friendly and visually appealing. It includes styles for the container, labels, input fields, button, and result display.
JavaScript Calculation
The JavaScript function calculatePension()
performs the pension calculation. It retrieves the user input values, calculates the number of years until retirement, and uses a loop to calculate the total savings at retirement. The result is then displayed in the div
element with the ID result
.
Customizing the Pension Calculator
You can customize the pension calculator to better suit your needs or the needs of your audience. Here are a few suggestions:
1. Adjust Input Limits
You can modify the min
and max
attributes of the input fields to set appropriate limits for age, retirement age, current savings, monthly contributions, and expected annual return.
<input type="number" id="age" min="18" max="100" value="30">
<input type="number" id="retirementAge" min="50" max="100" value="65">
<input type="number" id="currentSavings" min="0" value="10000">
<input type="number" id="monthlyContribution" min="0" value="200">
<input type="number" id="annualReturn" min="0" max="100" value="5">
2. Modify Styles
You can update the CSS styles to match your website’s design. Adjust colors, fonts, padding, margins, and other style properties as needed.
.calculator-container {
max-width: 400px;
margin: auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 10px;
background-color: #f9f9f9;
}
.calculator-container h2 {
text-align: center;
}
.calculator-container label {
display: block;
margin: 15px 0 5px;
}
.calculator-container input {
width: 100%;
padding: 10px;
margin-bottom: 10px;
border: 1px solid #ccc;
border-radius: 5px;
}
.calculator-container button {
width: 100%;
padding: 10px;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
.calculator-container button:hover {
background-color: #45a049;
}
.calculator-container .result {
margin-top: 20px;
padding: 10px;
border: 1px solid #ccc;
border-radius: 5px;
background-color: #e9e9e9;
text-align: center;
}
3. Enhance Functionality
Consider adding more advanced features to the calculator, such as additional input fields for inflation rate, tax considerations, or different investment scenarios.
Conclusion
By following the steps outlined in this guide, you can create a simple and effective pension calculator for your WordPress page. This tool can provide significant value to your visitors by helping them plan for their financial future. The provided HTML, CSS, and JavaScript code is easy to implement and customize, allowing you to tailor the calculator to your specific needs.
Embedding a pension calculator on your WordPress page not only enhances user engagement but also demonstrates your commitment to providing useful resources for financial planning. Encourage your visitors to use the calculator regularly and adjust their savings strategy based on the results.
Remember, the key to successful retirement planning is consistent saving and smart investment decisions. With this pension calculator, you can empower your users to take control of their financial future.