Reading time: 3 minutes.
Ensuring the accuracy of text input is crucial. So why not create a JS-based spell-checker? (Even if it’s just for fun.)
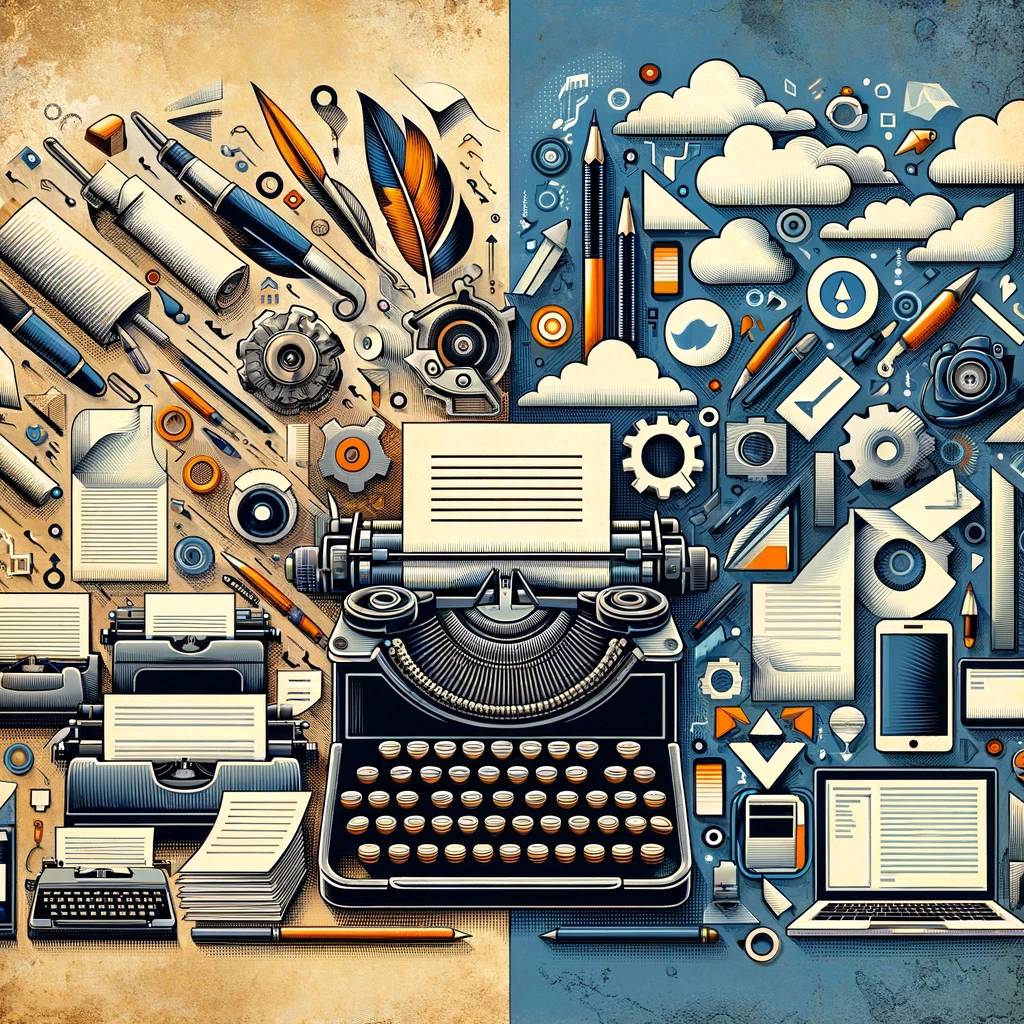
Whether it’s a blog, a social media post, or an official document, errors in spelling can detract from the message and professionalism of the content. JavaScript, being one of the most popular programming languages for web development, offers various ways to implement a spell-checker. In this article, we will explore how to create a basic JavaScript spell-checker.
Understanding the Basics
A spell-checker is a tool that compares words in a text against a dictionary of words and flags any word not found in the dictionary as a potential misspelling. The key components of a spell-checker are:
- Text Input: The text to be checked.
- Dictionary: A list of correctly spelled words.
- Algorithm: The logic to compare the text against the dictionary.
Setting Up the Environment
Firstly, ensure that you have a basic HTML and JavaScript setup. Create an HTML file (index.html
) and link it to a JavaScript file (spellchecker.js
).
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Spell Checker</title>
</head>
<body>
<textarea id="text-input" rows="10" cols="50"></textarea>
<button onclick="checkSpelling()">Check Spelling</button>
<div id="results"></div>
<script src="spellchecker.js"></script>
</body>
</html>
Building the Spell-Checker in JavaScript
spellchecker.js
First, we need to create a basic dictionary. For simplicity, we’ll use a small array of words. In a real-world application, this dictionary would be much larger, possibly sourced from a database or a large file (such as this one found on a GitHub.)
const dictionary = ['the', 'quick', 'brown', 'fox', 'jumps', 'over', 'lazy', 'dog'];
Next, we need a function to check the spelling.
function checkSpelling() {
let textInput = document.getElementById('text-input').value;
let words = textInput.split(' ');
let misspelledWords = [];
for (let word of words) {
if (!dictionary.includes(word)) {
misspelledWords.push(word);
}
}
displayResults(misspelledWords);
}
Here, we split the input text into words and check each word against the dictionary. If a word is not found in the dictionary, it’s added to the misspelledWords
array.
Finally, we need a function to display the results.
function displayResults(misspelledWords) {
let resultsDiv = document.getElementById('results');
if (misspelledWords.length > 0) {
resultsDiv.innerHTML = 'Misspelled Words: ' + misspelledWords.join(', ');
} else {
resultsDiv.innerHTML = 'No misspellings found!';
}
}
Enhancing the Spell-Checker
While the above spell-checker works for basic scenarios, it has limitations. It doesn’t handle case sensitivity, punctuation, or more complex error types like repeated letters or typos. Let’s enhance it.
Handling Case Sensitivity and Punctuation
Modify the checkSpelling
function to convert words to lower case and remove punctuation.
function cleanWord(word) {
return word.toLowerCase().replace(/[.,\/#!$%\^&\*;:{}=\-_`~()]/g,"");
}
function checkSpelling() {
// ... previous code ...
for (let word of words) {
let cleanWord = cleanWord(word);
if (!dictionary.includes(cleanWord)) {
misspelledWords.push(word);
}
}
// ... previous code ...
}
Expanding the Dictionary
In a real-world application, you might want to use a large dictionary. Consider loading a comprehensive list of words asynchronously, perhaps from a JSON file or an API.
let dictionary = [];
fetch('path/to/dictionary.json')
.then(response => response.json())
.then(data => {
dictionary = data.words;
});
Offering Suggestions
A useful feature of spell-checkers is suggesting correct spellings for misspelled words. Implementing a full-fledged suggestion algorithm is complex and beyond the scope of this article, but we can demonstrate a simple approach based on Levenshtein distance, which measures the number of edits needed to change one word into another.
You can use a library like fast-levenshtein
to calculate the distance and suggest words with the smallest distance to the misspelled word. (You might find one to suit your needs on this GitHub.)
import levenshtein from 'fast-levenshtein';
function getSuggestions(word) {
let suggestions = dictionary
.filter(dictWord =>
levenshtein.get(word, dictWord) < 3);
return suggestions;
}
Conclusion
Building a spell-checker in JavaScript is a task that can range from very simple to quite complex depending on the requirements. The basic version can be enhanced with case sensitivity handling, punctuation removal, a larger dictionary, and even suggestions for misspelled words.
Remember, spell-checking is a challenging area in natural language processing, and while a simple implementation can catch basic errors, more advanced features like contextual spelling checks (e.g., ‘their’ vs ‘there’) require more sophisticated approaches, often involving machine learning techniques.
This article provides a foundation for creating a JavaScript spell-checker and can be a starting point for more advanced text-processing tools. Keep exploring and enhancing your spell-checker to make it more robust and user-friendly. Happy coding!